ESP-MESH Programming Guide¶
This is a programming guide for ESP-MESH, including the API reference and coding examples. This guide is split into the following parts:
- ESP-MESH Programming Model
- Writing an ESP-MESH Application
- Self Organized Networking
- Application Examples
- API Reference
For documentation regarding the ESP-MESH protocol, please see the ESP-MESH API Guide.
ESP-MESH Programming Model¶
Software Stack¶
The ESP-MESH software stack is built atop the Wi-Fi Driver/FreeRTOS and may use the LwIP Stack in some instances (i.e. the root node). The following diagram illustrates the ESP-MESH software stack.
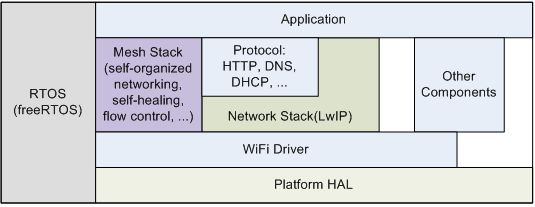
ESP-MESH Software Stack
System Events¶
An application interfaces with ESP-MESH via ESP-MESH Events. Since ESP-MESH is built atop the Wi-Fi stack, it is also possible for the application to interface with the Wi-Fi driver via the Wi-Fi Event Task. The following diagram illustrates the interfaces for the various System Events in an ESP-MESH application.
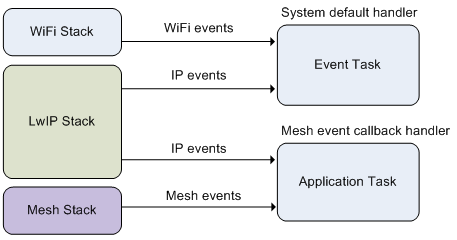
ESP-MESH System Events Delivery
The mesh_event_id_t
defines all possible ESP-MESH system events and can indicate events such as the connection/disconnection of parent/child. Before ESP-MESH system events can be used, the application must register a Mesh Event Callback via esp_mesh_set_config()
. The callback is used to receive events from the ESP-MESH stack as well as the LwIP Stack and should contain handlers for each event relevant to the application.
Typical use cases of system events include using events such as MESH_EVENT_PARENT_CONNECTED
and MESH_EVENT_CHILD_CONNECTED
to indicate when a node can begin transmitting data upstream and downstream respectively. Likewise, MESH_EVENT_ROOT_GOT_IP
and MESH_EVENT_ROOT_LOST_IP
can be used to indicate when the root node can and cannot transmit data to the external IP network.
Warning
When using ESP-MESH under self-organized mode, users must ensure that no calls to Wi-Fi API are made. This is due to the fact that the self-organizing mode will internally make Wi-Fi API calls to connect/disconnect/scan etc. Any Wi-Fi calls from the application (including calls from callbacks and handlers of Wi-Fi events) may interfere with ESP-MESH’s self-organizing behavior. Therefore, user’s should not call Wi-Fi APIs after esp_mesh_start()
is called, and before esp_mesh_stop()
is called.
LwIP & ESP-MESH¶
The application can access the ESP-MESH stack directly without having to go through the LwIP stack. The LwIP stack is only required by the root node to transmit/receive data to/from an external IP network. However, since every node can potentially become the root node (due to automatic root node selection), each node must still initialize the LwIP stack.
Each node is required to initialize LwIP by calling tcpip_adapter_init()
. In order to prevent non-root node access to LwIP, the application should stop the following services after LwIP initialization:
- DHCP server service on the softAP interface.
- DHCP client service on the station interface.
The following code snippet demonstrates how to initialize LwIP for ESP-MESH applications.
/* tcpip initialization */
tcpip_adapter_init();
/*
* for mesh
* stop DHCP server on softAP interface by default
* stop DHCP client on station interface by default
*/
ESP_ERROR_CHECK(tcpip_adapter_dhcps_stop(TCPIP_ADAPTER_IF_AP));
ESP_ERROR_CHECK(tcpip_adapter_dhcpc_stop(TCPIP_ADAPTER_IF_STA));
/* do not specify system event callback, use NULL instead. */
ESP_ERROR_CHECK(esp_event_loop_init(NULL, NULL));
Note
ESP-MESH requires a root node to be connected with a router. Therefore, in the event that a node becomes the root, the corresponding handler must start the DHCP client service and immediately obtain an IP address. Doing so will allow other nodes to begin transmitting/receiving packets to/from the external IP network. However, this step is unnecessary if static IP settings are used.
Writing an ESP-MESH Application¶
The prerequisites for starting ESP-MESH is to initialize LwIP and Wi-Fi, The following code snippet demonstrates the necessary prerequisite steps before ESP-MESH itself can be initialized.
tcpip_adapter_init();
/*
* for mesh
* stop DHCP server on softAP interface by default
* stop DHCP client on station interface by default
*/
ESP_ERROR_CHECK(tcpip_adapter_dhcps_stop(TCPIP_ADAPTER_IF_AP));
ESP_ERROR_CHECK(tcpip_adapter_dhcpc_stop(TCPIP_ADAPTER_IF_STA));
/* do not specify system event callback, use NULL instead. */
ESP_ERROR_CHECK(esp_event_loop_init(NULL, NULL));
/* Wi-Fi initialization */
wifi_init_config_t config = WIFI_INIT_CONFIG_DEFAULT();
ESP_ERROR_CHECK(esp_wifi_init(&config));
ESP_ERROR_CHECK(esp_wifi_set_storage(WIFI_STORAGE_FLASH));
ESP_ERROR_CHECK(esp_wifi_start());
After initializing LwIP and Wi-Fi, the process of getting an ESP-MESH network up and running can be summarized into the following three steps:
Initialize Mesh¶
The following code snippet demonstrates how to initialize ESP-MESH
/* mesh initialization */
ESP_ERROR_CHECK(esp_mesh_init());
Configuring an ESP-MESH Network¶
ESP-MESH is configured via esp_mesh_set_config()
which receives its arguments using the mesh_cfg_t
structure. The structure contains the following parameters used to configure ESP-MESH:
Parameter | Description |
---|---|
Channel | Range from 1 to 14 |
Event Callback | Callback for Mesh Events,
see mesh_event_cb_t |
Mesh ID | ID of ESP-MESH Network,
see mesh_addr_t |
Router | Router Configuration,
see mesh_router_t |
Mesh AP | Mesh AP Configuration,
see mesh_ap_cfg_t |
Crypto Functions | Crypto Functions for Mesh IE,
see mesh_crypto_funcs_t |
The following code snippet demonstrates how to configure ESP-MESH.
/* Enable the Mesh IE encryption by default */
mesh_cfg_t cfg = MESH_INIT_CONFIG_DEFAULT();
/* mesh ID */
memcpy((uint8_t *) &cfg.mesh_id, MESH_ID, 6);
/* mesh event callback */
cfg.event_cb = &mesh_event_handler;
/* channel (must match the router's channel) */
cfg.channel = CONFIG_MESH_CHANNEL;
/* router */
cfg.router.ssid_len = strlen(CONFIG_MESH_ROUTER_SSID);
memcpy((uint8_t *) &cfg.router.ssid, CONFIG_MESH_ROUTER_SSID, cfg.router.ssid_len);
memcpy((uint8_t *) &cfg.router.password, CONFIG_MESH_ROUTER_PASSWD,
strlen(CONFIG_MESH_ROUTER_PASSWD));
/* mesh softAP */
cfg.mesh_ap.max_connection = CONFIG_MESH_AP_CONNECTIONS;
memcpy((uint8_t *) &cfg.mesh_ap.password, CONFIG_MESH_AP_PASSWD,
strlen(CONFIG_MESH_AP_PASSWD));
ESP_ERROR_CHECK(esp_mesh_set_config(&cfg));
Start Mesh¶
The following code snippet demonstrates how to start ESP-MESH.
/* mesh start */
ESP_ERROR_CHECK(esp_mesh_start());
After starting ESP-MESH, the application should check for ESP-MESH events to determine when it has connected to the network. After connecting, the application can start transmitting and receiving packets over the ESP-MESH network using esp_mesh_send()
and esp_mesh_recv()
.
Self Organized Networking¶
Self organized networking is a feature of ESP-MESH where nodes can autonomously scan/select/connect/reconnect to other nodes and routers. This feature allows an ESP-MESH network to operate with high degree of autonomy by making the network robust to dynamic network topologies and conditions. With self organized networking enabled, nodes in an ESP-MESH network are able to carryout the following actions without autonomously:
- Selection or election of the root node (see Automatic Root Node Selection in Building a Network)
- Selection of a preferred parent node (see Parent Node Selection in Building a Network)
- Automatic reconnection upon detecting a disconnection (see Intermediate Parent Node Failure in Managing a Network)
When self organized networking is enabled, the ESP-MESH stack will internally make calls to Wi-Fi driver APIs. Therefore, the application layer should not make any calls to Wi-Fi driver APIs whilst self organized networking is enabled as doing so would risk interfering with ESP-MESH.
Toggling Self Organized Networking¶
Self organized networking can be enabled or disabled by the application at runtime by calling the esp_mesh_set_self_organized()
function. The function has the two following parameters:
bool enable
specifies whether to enable or disable self organized networking.bool select_parent
specifies whether a new parent node should be selected when enabling self organized networking. Selecting a new parent has different effects depending the node type and the node’s current state. This parameter is unused when disabling self organized networking.
Disabling Self Organized Networking¶
The following code snippet demonstrates how to disable self organized networking.
//Disable self organized networking
esp_mesh_set_self_organized(false, false);
ESP-MESH will attempt to maintain the node’s current Wi-Fi state when disabling self organized networking.
- If the node was previously connected to other nodes, it will remain connected.
- If the node was previously disconnected and was scanning for a parent node or router, it will stop scanning.
- If the node was previously attempting to reconnect to a parent node or router, it will stop reconnecting.
Enabling Self Organized Networking¶
ESP-MESH will attempt to maintain the node’s current Wi-Fi state when enabling self organized networking. However, depending on the node type and whether a new parent is selected, the Wi-Fi state of the node can change. The following table shows effects of enabling self organized networking.
Select Parent | Is Root Node | Effects |
---|---|---|
N | N |
|
Y |
|
|
Y | N |
|
Y |
|
The following code snipping demonstrates how to enable self organized networking.
//Enable self organized networking and select a new parent
esp_mesh_set_self_organized(true, true);
...
//Enable self organized networking and manually reconnect
esp_mesh_set_self_organized(true, false);
esp_mesh_connect();
Calling Wi-Fi Driver API¶
There can be instances in which an application may want to directly call Wi-Fi driver API whilst using ESP-MESH. For example, an application may want to manually scan for neighboring APs. However, self organized networking must be disabled before the application calls any Wi-Fi driver APIs. This will prevent the ESP-MESH stack from attempting to call any Wi-Fi driver APIs and potentially interfering with the application’s calls.
Therefore, application calls to Wi-Fi driver APIs should be placed in between calls of esp_mesh_set_self_organized()
which disable and enable self organized networking. The following code snippet demonstrates how an application can safely call esp_wifi_scan_start()
whilst using ESP-MESH.
//Disable self organized networking
esp_mesh_set_self_organized(0, 0);
//Stop any scans already in progress
esp_wifi_scan_stop();
//Manually start scan. Will automatically stop when run to completion
esp_wifi_scan_start();
//Process scan results
...
//Re-enable self organized networking if still connected
esp_mesh_set_self_organized(1, 0);
...
//Re-enable self organized networking if non-root and disconnected
esp_mesh_set_self_organized(1, 1);
...
//Re-enable self organized networking if root and disconnected
esp_mesh_set_self_organized(1, 0); //Don't select new parent
esp_mesh_connect(); //Manually reconnect to router
Application Examples¶
ESP-IDF contains these ESP-MESH example projects:
The Internal Communication Example demonstrates how to setup a ESP-MESH network and have the root node send a data packet to every node within the network.
The Manual Networking Example demonstrates how to use ESP-MESH without the self-organizing features. This example shows how to program a node to manually scan for a list of potential parent nodes and select a parent node based on custom criteria.
API Reference¶
Header File¶
Functions¶
-
esp_err_t
esp_mesh_init
(void)¶ Mesh initialization.
- Check whether Wi-Fi is started.
- Initialize mesh global variables with default values.
- Attention
- This API shall be called after Wi-Fi is started.
- Return
- ESP_OK
- ESP_FAIL
-
esp_err_t
esp_mesh_deinit
(void)¶ Mesh de-initialization.
- Release resources and stop the mesh
- Return
- ESP_OK
- ESP_FAIL
-
esp_err_t
esp_mesh_start
(void)¶ Start mesh.
- Initialize mesh IE.
- Start mesh network management service.
- Create TX and RX queues according to the configuration.
- Register mesh packets receive callback.
- Attention
- This API shall be called after mesh initialization and configuration.
- Return
- ESP_OK
- ESP_FAIL
- ESP_ERR_MESH_NOT_INIT
- ESP_ERR_MESH_NOT_CONFIG
- ESP_ERR_MESH_NO_MEMORY
-
esp_err_t
esp_mesh_stop
(void)¶ Stop mesh.
- Deinitialize mesh IE.
- Disconnect with current parent.
- Disassociate all currently associated children.
- Stop mesh network management service.
- Unregister mesh packets receive callback.
- Delete TX and RX queues.
- Release resources.
- Restore Wi-Fi softAP to default settings if Wi-Fi dual mode is enabled.
- Return
- ESP_OK
- ESP_FAIL
-
esp_err_t
esp_mesh_send
(const mesh_addr_t *to, const mesh_data_t *data, int flag, const mesh_opt_t opt[], int opt_count)¶ Send a packet over the mesh network.
- Send a packet to any device in the mesh network.
- Send a packet to external IP network.
- Attention
- This API is not reentrant.
- Return
- ESP_OK
- ESP_FAIL
- ESP_ERR_MESH_ARGUMENT
- ESP_ERR_MESH_NOT_START
- ESP_ERR_MESH_DISCONNECTED
- ESP_ERR_MESH_OPT_UNKNOWN
- ESP_ERR_MESH_EXCEED_MTU
- ESP_ERR_MESH_NO_MEMORY
- ESP_ERR_MESH_TIMEOUT
- ESP_ERR_MESH_QUEUE_FULL
- ESP_ERR_MESH_NO_ROUTE_FOUND
- ESP_ERR_MESH_DISCARD
- Parameters
to
: the address of the final destination of the packet- If the packet is to the root, set this parameter to NULL.
- If the packet is to an external IP network, set this parameter to the IPv4:PORT combination. This packet will be delivered to the root firstly, then the root will forward this packet to the final IP server address.
data
: pointer to a sending mesh packet- Field size should not exceed MESH_MPS. Note that the size of one mesh packet should not exceed MESH_MTU.
- Field proto should be set to data protocol in use (default is MESH_PROTO_BIN for binary).
- Field tos should be set to transmission tos (type of service) in use (default is MESH_TOS_P2P for point-to-point reliable).
flag
: bitmap for data sent- Speed up the route search
- If the packet is to the root and “to” parameter is NULL, set this parameter to 0.
- If the packet is to an internal device, MESH_DATA_P2P should be set.
- If the packet is to the root (“to” parameter isn’t NULL) or to external IP network, MESH_DATA_TODS should be set.
- If the packet is from the root to an internal device, MESH_DATA_FROMDS should be set.
- Specify whether this API is block or non-block, block by default
- If needs non-block, MESH_DATA_NONBLOCK should be set.
- In the situation of the root change, MESH_DATA_DROP identifies this packet can be dropped by the new root for upstream data to external IP network, we try our best to avoid data loss caused by the root change, but there is a risk that the new root is running out of memory because most of memory is occupied by the pending data which isn’t read out in time by esp_mesh_recv_toDS().
Generally, we suggest esp_mesh_recv_toDS() is called after a connection with IP network is created. Thus data outgoing to external IP network via socket is just from reading esp_mesh_recv_toDS() which avoids unnecessary memory copy.
- Speed up the route search
opt
: options- In case of sending a packet to a certain group, MESH_OPT_SEND_GROUP is a good choice. In this option, the value field should be set to the target receiver addresses in this group.
- Root sends a packet to an internal device, this packet is from external IP network in case the receiver device responds this packet, MESH_OPT_RECV_DS_ADDR is required to attach the target DS address.
opt_count
: option count- Currently, this API only takes one option, so opt_count is only supported to be 1.
-
esp_err_t
esp_mesh_recv
(mesh_addr_t *from, mesh_data_t *data, int timeout_ms, int *flag, mesh_opt_t opt[], int opt_count)¶ Receive a packet targeted to self over the mesh network.
flag could be MESH_DATA_FROMDS or MESH_DATA_TODS.
- Attention
- Mesh RX queue should be checked regularly to avoid running out of memory.
- Use esp_mesh_get_rx_pending() to check the number of packets available in the queue waiting to be received by applications.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- ESP_ERR_MESH_NOT_START
- ESP_ERR_MESH_TIMEOUT
- ESP_ERR_MESH_DISCARD
- Parameters
from
: the address of the original source of the packetdata
: pointer to the received mesh packet- Field proto is the data protocol in use. Should follow it to parse the received data.
- Field tos is the transmission tos (type of service) in use.
timeout_ms
: wait time if a packet isn’t immediately available (0:no wait, portMAX_DELAY:wait forever)flag
: bitmap for data received- MESH_DATA_FROMDS represents data from external IP network
- MESH_DATA_TODS represents data directed upward within the mesh network
- Parameters
opt
: options desired to receive- MESH_OPT_RECV_DS_ADDR attaches the DS address
opt_count
: option count desired to receive- Currently, this API only takes one option, so opt_count is only supported to be 1.
-
esp_err_t
esp_mesh_recv_toDS
(mesh_addr_t *from, mesh_addr_t *to, mesh_data_t *data, int timeout_ms, int *flag, mesh_opt_t opt[], int opt_count)¶ Receive a packet targeted to external IP network.
- Root uses this API to receive packets destined to external IP network
- Root forwards the received packets to the final destination via socket.
- If no socket connection is ready to send out the received packets and this esp_mesh_recv_toDS() hasn’t been called by applications, packets from the whole mesh network will be pending in toDS queue.
Use esp_mesh_get_rx_pending() to check the number of packets available in the queue waiting to be received by applications in case of running out of memory in the root.
Using esp_mesh_set_xon_qsize() users may configure the RX queue size, default:32. If this size is too large, and esp_mesh_recv_toDS() isn’t called in time, there is a risk that a great deal of memory is occupied by the pending packets. If this size is too small, it will impact the efficiency on upstream. How to decide this value depends on the specific application scenarios.
flag could be MESH_DATA_TODS.
- Attention
- This API is only called by the root.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- ESP_ERR_MESH_NOT_START
- ESP_ERR_MESH_TIMEOUT
- ESP_ERR_MESH_DISCARD
- Parameters
from
: the address of the original source of the packetto
: the address contains remote IP address and port (IPv4:PORT)data
: pointer to the received packet- Contain the protocol and applications should follow it to parse the data.
timeout_ms
: wait time if a packet isn’t immediately available (0:no wait, portMAX_DELAY:wait forever)flag
: bitmap for data received- MESH_DATA_TODS represents the received data target to external IP network. Root shall forward this data to external IP network via the association with router.
- Parameters
opt
: options desired to receiveopt_count
: option count desired to receive
-
esp_err_t
esp_mesh_set_config
(const mesh_cfg_t *config)¶ Set mesh stack configuration.
- Use MESH_INIT_CONFIG_DEFAULT() to initialize the default values, mesh IE is encrypted by default.
- Mesh network is established on a fixed channel (1-14).
- Mesh event callback is mandatory.
- Mesh ID is an identifier of an MBSS. Nodes with the same mesh ID can communicate with each other.
- Regarding to the router configuration, if the router is hidden, BSSID field is mandatory.
If BSSID field isn’t set and there exists more than one router with same SSID, there is a risk that more roots than one connected with different BSSID will appear. It means more than one mesh network is established with the same mesh ID.
Root conflict function could eliminate redundant roots connected with the same BSSID, but couldn’t handle roots connected with different BSSID. Because users might have such requirements of setting up routers with same SSID for the future replacement. But in that case, if the above situations happen, please make sure applications implement forward functions on the root to guarantee devices in different mesh networks can communicate with each other. max_connection of mesh softAP is limited by the max number of Wi-Fi softAP supported (max:10).
- Attention
- This API shall be called before mesh is started after mesh is initialized.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- ESP_ERR_MESH_NOT_ALLOWED
- Parameters
config
: pointer to mesh stack configuration
-
esp_err_t
esp_mesh_get_config
(mesh_cfg_t *config)¶ Get mesh stack configuration.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- Parameters
config
: pointer to mesh stack configuration
-
esp_err_t
esp_mesh_set_router
(const mesh_router_t *router)¶ Get router configuration.
- Attention
- This API is used to dynamically modify the router configuration after mesh is configured.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- Parameters
router
: pointer to router configuration
-
esp_err_t
esp_mesh_get_router
(mesh_router_t *router)¶ Get router configuration.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- Parameters
router
: pointer to router configuration
-
esp_err_t
esp_mesh_set_id
(const mesh_addr_t *id)¶ Set mesh network ID.
- Attention
- This API is used to dynamically modify the mesh network ID.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT: invalid argument
- Parameters
id
: pointer to mesh network ID
-
esp_err_t
esp_mesh_get_id
(mesh_addr_t *id)¶ Get mesh network ID.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- Parameters
id
: pointer to mesh network ID
-
esp_err_t
esp_mesh_set_type
(mesh_type_t type)¶ Designate device type over the mesh network.
- MESH_ROOT: designates the root node for a mesh network
- MESH_LEAF: designates a device as a standalone Wi-Fi station
- Return
- ESP_OK
- ESP_ERR_MESH_NOT_ALLOWED
- Parameters
type
: device type
-
mesh_type_t
esp_mesh_get_type
(void)¶ Get device type over mesh network.
- Attention
- This API shall be called after having received the event MESH_EVENT_PARENT_CONNECTED.
- Return
- mesh type
-
esp_err_t
esp_mesh_set_max_layer
(int max_layer)¶ Set network max layer value (max:25, default:25)
- Network max layer limits the max hop count.
- Attention
- This API shall be called before mesh is started.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- ESP_ERR_MESH_NOT_ALLOWED
- Parameters
max_layer
: max layer value
-
int
esp_mesh_get_max_layer
(void)¶ Get max layer value.
- Return
- max layer value
-
esp_err_t
esp_mesh_set_ap_password
(const uint8_t *pwd, int len)¶ Set mesh softAP password.
- Attention
- This API shall be called before mesh is started.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- ESP_ERR_MESH_NOT_ALLOWED
- Parameters
pwd
: pointer to the passwordlen
: password length
-
esp_err_t
esp_mesh_set_ap_authmode
(wifi_auth_mode_t authmode)¶ Set mesh softAP authentication mode.
- Attention
- This API shall be called before mesh is started.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- ESP_ERR_MESH_NOT_ALLOWED
- Parameters
authmode
: authentication mode
-
wifi_auth_mode_t
esp_mesh_get_ap_authmode
(void)¶ Get mesh softAP authentication mode.
- Return
- authentication mode
-
esp_err_t
esp_mesh_set_ap_connections
(int connections)¶ Set mesh softAP max connection value.
- Attention
- This API shall be called before mesh is started.
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- Parameters
connections
: the number of max connections
-
int
esp_mesh_get_ap_connections
(void)¶ Get mesh softAP max connection configuration.
- Return
- the number of max connections
-
int
esp_mesh_get_layer
(void)¶ Get current layer value over the mesh network.
- Attention
- This API shall be called after having received the event MESH_EVENT_PARENT_CONNECTED.
- Return
- layer value
-
esp_err_t
esp_mesh_get_parent_bssid
(mesh_addr_t *bssid)¶ Get the parent BSSID.
- Attention
- This API shall be called after having received the event MESH_EVENT_PARENT_CONNECTED.
- Return
- ESP_OK
- ESP_FAIL
- Parameters
bssid
: pointer to parent BSSID
-
bool
esp_mesh_is_root
(void)¶ Return whether the device is the root node of the network.
- Return
- true/false
-
esp_err_t
esp_mesh_set_self_organized
(bool enable, bool select_parent)¶ Enable/disable self-organized networking.
- Self-organized networking has three main functions: select the root node; find a preferred parent; initiate reconnection if a disconnection is detected.
- Self-organized networking is enabled by default.
- If self-organized is disabled, users should set a parent for the device via esp_mesh_set_parent().
- Attention
- This API is used to dynamically modify whether to enable the self organizing.
- Return
- ESP_OK
- ESP_FAIL
- Parameters
enable
: enable or disable self-organized networkingselect_parent
: Only valid when self-organized networking is enabled.- if select_parent is set to true, the root will give up its mesh root status and search for a new parent like other non-root devices.
-
bool
esp_mesh_get_self_organized
(void)¶ Return whether enable self-organized networking or not.
- Return
- true/false
-
esp_err_t
esp_mesh_waive_root
(const mesh_vote_t *vote, int reason)¶ Cause the root device to give up (waive) its mesh root status.
- A device is elected root primarily based on RSSI from the external router.
- If external router conditions change, users can call this API to perform a root switch.
- In this API, users could specify a desired root address to replace itself or specify an attempts value to ask current root to initiate a new round of voting. During the voting, a better root candidate would be expected to find to replace the current one.
- If no desired root candidate, the vote will try a specified number of attempts (at least 15). If no better root candidate is found, keep the current one. If a better candidate is found, the new better one will send a root switch request to the current root, current root will respond with a root switch acknowledgment.
- After that, the new candidate will connect to the router to be a new root, the previous root will disconnect with the router and choose another parent instead.
Root switch is completed with minimal disruption to the whole mesh network.
- Attention
- This API is only called by the root.
- Return
- ESP_OK
- ESP_ERR_MESH_QUEUE_FULL
- ESP_ERR_MESH_DISCARD
- ESP_FAIL
- Parameters
vote
: vote configuration- If this parameter is set NULL, the vote will perform the default 15 times.
- Field percentage threshold is 0.9 by default.
- Field is_rc_specified shall be false.
- Field attempts shall be at least 15 times.
reason
: only accept MESH_VOTE_REASON_ROOT_INITIATED for now
-
esp_err_t
esp_mesh_set_vote_percentage
(float percentage)¶ Set vote percentage threshold for approval of being a root.
- During the networking, only obtaining vote percentage reaches this threshold, the device could be a root.
- Attention
- This API shall be called before mesh is started.
- Return
- ESP_OK
- ESP_FAIL
- Parameters
percentage
: vote percentage threshold
-
float
esp_mesh_get_vote_percentage
(void)¶ Get vote percentage threshold for approval of being a root.
- Return
- percentage threshold
-
esp_err_t
esp_mesh_set_ap_assoc_expire
(int seconds)¶ Set mesh softAP associate expired time (default:10 seconds)
- If mesh softAP hasn’t received any data from an associated child within this time, mesh softAP will take this child inactive and disassociate it.
- If mesh softAP is encrypted, this value should be set a greater value, such as 30 seconds.
- Return
- ESP_OK
- ESP_FAIL
- Parameters
seconds
: the expired time
-
int
esp_mesh_get_ap_assoc_expire
(void)¶ Get mesh softAP associate expired time.
- Return
- seconds
-
int
esp_mesh_get_total_node_num
(void)¶ Get total number of devices in current network (including the root)
- Attention
- The returned value might be incorrect when the network is changing.
- Return
- total number of devices (including the root)
-
int
esp_mesh_get_routing_table_size
(void)¶ Get the number of devices in this device’s sub-network (including self)
- Return
- the number of devices over this device’s sub-network (including self)
-
esp_err_t
esp_mesh_get_routing_table
(mesh_addr_t *mac, int len, int *size)¶ Get routing table of this device’s sub-network (including itself)
- Return
- ESP_OK
- ESP_ERR_MESH_ARGUMENT
- Parameters
mac
: pointer to routing tablelen
: routing table size(in bytes)size
: pointer to the number of devices in routing table (including itself)
-
esp_err_t
esp_mesh_post_toDS_state
(bool reachable)¶ Post the toDS state to the mesh stack.
- Attention
- This API is only for the root.
- Return
- ESP_OK
- ESP_FAIL
- Parameters
reachable
: this state represents whether the root is able to access external IP network
-
esp_err_t
esp_mesh_get_tx_pending
(mesh_tx_pending_t *pending)¶ Return the number of packets pending in the queue waiting to be sent by the mesh stack.
- Return
- ESP_OK
- ESP_FAIL
- Parameters
pending
: pointer to the TX pending
-
esp_err_t
esp_mesh_get_rx_pending
(mesh_rx_pending_t *pending)¶ Return the number of packets available in the queue waiting to be received by applications.
- Return
- ESP_OK
- ESP_FAIL
- Parameters
pending
: pointer to the RX pending
-
int
esp_mesh_available_txupQ_num
(const mesh_addr_t *addr, uint32_t *xseqno_in)¶ Return the number of packets could be accepted from the specified address.
- Return
- the number of upQ for a certain address
- Parameters
addr
: self address or an associate children addressxseqno_in
: sequence number of the last received packet from the specified address
-
esp_err_t
esp_mesh_set_xon_qsize
(int qsize)¶ Set the number of queue.
- Attention
- This API shall be called before mesh is started.
- Return
- ESP_OK
- ESP_FAIL
- Parameters
qsize
: default:32 (min:16)
-
int
esp_mesh_get_xon_qsize
(void)¶ Get queue size.
- Return
- the number of queue
-
esp_err_t
esp_mesh_allow_root_conflicts
(bool allowed)¶ Set whether allow more than one root existing in one network.
- Return
- ESP_OK
- ESP_WIFI_ERR_NOT_INIT
- ESP_WIFI_ERR_NOT_START
- Parameters
allowed
: allow or not
-
bool
esp_mesh_is_root_conflicts_allowed
(void)¶ Check whether allow more than one root to exist in one network.
- Return
- true/false
-
esp_err_t
esp_mesh_set_group_id
(const mesh_addr_t *addr, int num)¶ Set group ID addresses.
- Return
- ESP_OK
- ESP_MESH_ERR_ARGUMENT
- Parameters
addr
: pointer to new group ID addressesnum
: the number of group ID addresses
-
esp_err_t
esp_mesh_delete_group_id
(const mesh_addr_t *addr, int num)¶ Delete group ID addresses.
- Return
- ESP_OK
- ESP_MESH_ERR_ARGUMENT
- Parameters
addr
: pointer to deleted group ID addressnum
: the number of group ID addresses
-
int
esp_mesh_get_group_num
(void)¶ Get the number of group ID addresses.
- Return
- the number of group ID addresses
-
esp_err_t
esp_mesh_get_group_list
(mesh_addr_t *addr, int num)¶ Get group ID addresses.
- Return
- ESP_OK
- ESP_MESH_ERR_ARGUMENT
- Parameters
addr
: pointer to group ID addressesnum
: the number of group ID addresses
-
bool
esp_mesh_is_my_group
(const mesh_addr_t *addr)¶ Check whether the specified group address is my group.
- Return
- true/false
-
esp_err_t
esp_mesh_set_capacity_num
(int num)¶ Set mesh network capacity (max:1000, default:300)
- Attention
- This API shall be called before mesh is started.
- Return
- ESP_OK
- ESP_ERR_MESH_NOT_ALLOWED
- ESP_MESH_ERR_ARGUMENT
- Parameters
num
: mesh network capacity
-
int
esp_mesh_get_capacity_num
(void)¶ Get mesh network capacity.
- Return
- mesh network capacity
-
esp_err_t
esp_mesh_set_ie_crypto_funcs
(const mesh_crypto_funcs_t *crypto_funcs)¶ Set mesh IE crypto functions.
- Attention
- This API can be called at any time after mesh is initialized.
- Return
- ESP_OK
- Parameters
crypto_funcs
: crypto functions for mesh IE- If crypto_funcs is set to NULL, mesh IE is no longer encrypted.
-
esp_err_t
esp_mesh_set_ie_crypto_key
(const char *key, int len)¶ Set mesh IE crypto key.
- Attention
- This API can be called at any time after mesh is initialized.
- Return
- ESP_OK
- ESP_MESH_ERR_ARGUMENT
- Parameters
key
: ASCII crypto keylen
: length in bytes, range:8~64
-
esp_err_t
esp_mesh_get_ie_crypto_key
(char *key, int len)¶ Get mesh IE crypto key.
- Return
- ESP_OK
- ESP_MESH_ERR_ARGUMENT
- Parameters
key
: ASCII crypto keylen
: length in bytes, range:8~64
-
esp_err_t
esp_mesh_set_root_healing_delay
(int delay_ms)¶ Set delay time before starting root healing.
- Return
- ESP_OK
- Parameters
delay_ms
: delay time in milliseconds
-
int
esp_mesh_get_root_healing_delay
(void)¶ Get delay time before network starts root healing.
- Return
- delay time in milliseconds
-
esp_err_t
esp_mesh_set_event_cb
(const mesh_event_cb_t event_cb)¶ Set mesh event callback.
- Return
- ESP_OK
- Parameters
event_cb
: mesh event call back
-
esp_err_t
esp_mesh_fix_root
(bool enable)¶ Enable network Fixed Root Setting.
- Enabling fixed root disables automatic election of the root node via voting.
- All devices in the network shall use the same Fixed Root Setting (enabled or disabled).
- If Fixed Root is enabled, users should make sure a root node is designated for the network.
- Return
- ESP_OK
- Parameters
enable
: enable or not
-
bool
esp_mesh_is_root_fixed
(void)¶ Check whether network Fixed Root Setting is enabled.
- Enable/disable network Fixed Root Setting by API esp_mesh_fix_root().
- Network Fixed Root Setting also changes with the “flag” value in parent networking IE.
- Return
- true/false
-
esp_err_t
esp_mesh_set_parent
(const wifi_config_t *parent, const mesh_addr_t *parent_mesh_id, mesh_type_t my_type, int my_layer)¶ Set a specified parent for the device.
- Attention
- This API can be called at any time after mesh is configured.
- Return
- ESP_OK
- ESP_ERR_ARGUMENT
- ESP_ERR_MESH_NOT_CONFIG
- Parameters
parent
: parent configuration, the SSID and the channel of the parent are mandatory.- If the BSSID is set, make sure that the SSID and BSSID represent the same parent, otherwise the device will never find this specified parent.
parent_mesh_id
: parent mesh ID,- If this value is not set, the original mesh ID is used.
my_type
: mesh type- If the parent set for the device is the same as the router in the network configuration, then my_type shall set MESH_ROOT and my_layer shall set MESH_ROOT_LAYER.
my_layer
: mesh layer- my_layer of the device may change after joining the network.
- If my_type is set MESH_NODE, my_layer shall be greater than MESH_ROOT_LAYER.
- If my_type is set MESH_LEAF, the device becomes a standalone Wi-Fi station and no longer has the ability to extend the network.
-
esp_err_t
esp_mesh_scan_get_ap_ie_len
(int *len)¶ Get mesh networking IE length of one AP.
- Return
- ESP_OK
- ESP_ERR_WIFI_NOT_INIT
- ESP_ERR_WIFI_ARG
- ESP_ERR_WIFI_FAIL
- Parameters
len
: mesh networking IE length
-
esp_err_t
esp_mesh_scan_get_ap_record
(wifi_ap_record_t *ap_record, void *buffer)¶ Get AP record.
- Attention
- Different from esp_wifi_scan_get_ap_records(), this API only gets one of APs scanned each time. See “manual_networking” example.
- Return
- ESP_OK
- ESP_ERR_WIFI_NOT_INIT
- ESP_ERR_WIFI_ARG
- ESP_ERR_WIFI_FAIL
- Parameters
ap_record
: pointer to one AP recordbuffer
: pointer to the mesh networking IE of this AP
-
esp_err_t
esp_mesh_flush_upstream_packets
(void)¶ Flush upstream packets pending in to_parent queue and to_parent_p2p queue.
- Return
- ESP_OK
-
esp_err_t
esp_mesh_get_subnet_nodes_num
(const mesh_addr_t *child_mac, int *nodes_num)¶ Get the number of nodes in the subnet of a specific child.
- Return
- ESP_OK
- ESP_ERR_MESH_NOT_START
- ESP_ERR_MESH_ARGUMENT
- Parameters
child_mac
: an associated child address of this devicenodes_num
: pointer to the number of nodes in the subnet of a specific child
-
esp_err_t
esp_mesh_get_subnet_nodes_list
(const mesh_addr_t *child_mac, mesh_addr_t *nodes, int nodes_num)¶ Get nodes in the subnet of a specific child.
- Return
- ESP_OK
- ESP_ERR_MESH_NOT_START
- ESP_ERR_MESH_ARGUMENT
- Parameters
child_mac
: an associated child address of this devicenodes
: pointer to nodes in the subnet of a specific childnodes_num
: the number of nodes in the subnet of a specific child
-
esp_err_t
esp_mesh_switch_channel
(const uint8_t *new_bssid, int csa_newchan, int csa_count)¶ Cause the root device to add Channel Switch Announcement Element (CSA IE) to beacon.
- Set the new channel
- Set how many beacons with CSA IE will be sent before changing a new channel
- Enable the channel switch function
- Attention
- This API is only called by the root.
- Return
- ESP_OK
- Parameters
new_bssid
: the new router BSSID if the router changescsa_newchan
: the new channel number to which the whole network is movingcsa_count
: channel switch period(beacon count), unit is based on beacon interval of its softAP, the default value is 15.
-
esp_err_t
esp_mesh_get_router_bssid
(uint8_t *router_bssid)¶ Get the router BSSID.
- Return
- ESP_OK
- ESP_ERR_WIFI_NOT_INIT
- ESP_ERR_WIFI_ARG
- Parameters
router_bssid
: pointer to the router BSSID
-
int64_t
esp_mesh_get_tsf_time
(void)¶ Get the TSF time.
- Return
- the TSF time
Unions¶
-
union
mesh_addr_t
¶ - #include <esp_mesh.h>
Mesh address.
-
union
mesh_event_info_t
¶ - #include <esp_mesh.h>
Mesh event information.
Public Members
-
mesh_event_channel_switch_t
channel_switch
¶ channel switch
-
mesh_event_child_connected_t
child_connected
¶ child connected
-
mesh_event_child_disconnected_t
child_disconnected
¶ child disconnected
-
mesh_event_routing_table_change_t
routing_table
¶ routing table change
-
mesh_event_connected_t
connected
¶ parent connected
-
mesh_event_disconnected_t
disconnected
¶ parent disconnected
-
mesh_event_no_parent_found_t
no_parent
¶ no parent found
-
mesh_event_layer_change_t
layer_change
¶ layer change
-
mesh_event_toDS_state_t
toDS_state
¶ toDS state, devices shall check this state firstly before trying to send packets to external IP network. This state indicates right now whether the root is capable of sending packets out. If not, devices had better to wait until this state changes to be MESH_TODS_REACHABLE.
-
mesh_event_vote_started_t
vote_started
¶ vote started
-
mesh_event_root_got_ip_t
got_ip
¶ root obtains IP address
-
mesh_event_root_address_t
root_addr
¶ root address
-
mesh_event_root_switch_req_t
switch_req
¶ root switch request
-
mesh_event_root_conflict_t
root_conflict
¶ other powerful root
-
mesh_event_root_fixed_t
root_fixed
¶ fixed root
-
mesh_event_scan_done_t
scan_done
¶ scan done
-
mesh_event_network_state_t
network_state
¶ network state, such as whether current mesh network has a root.
-
mesh_event_find_network_t
find_network
¶ network found that can join
-
mesh_event_router_switch_t
router_switch
¶ new router information
-
mesh_event_channel_switch_t
-
union
mesh_rc_config_t
¶ - #include <esp_mesh.h>
Vote address configuration.
Public Members
-
int
attempts
¶ max vote attempts before a new root is elected automatically by mesh network. (min:15, 15 by default)
-
mesh_addr_t
rc_addr
¶ a new root address specified by users for API esp_mesh_waive_root()
-
int
Structures¶
-
struct
mip_t
¶ IP address and port.
-
struct
mesh_event_channel_switch_t
¶ Channel switch information.
Public Members
-
uint8_t
channel
¶ new channel
-
uint8_t
-
struct
mesh_event_connected_t
¶ Parent connected information.
-
struct
mesh_event_no_parent_found_t
¶ No parent found information.
Public Members
-
int
scan_times
¶ scan times being through
-
int
-
struct
mesh_event_layer_change_t
¶ Layer change information.
Public Members
-
uint8_t
new_layer
¶ new layer
-
uint8_t
-
struct
mesh_event_vote_started_t
¶ vote started information
Public Members
-
int
reason
¶ vote reason, vote could be initiated by children or by the root itself
-
int
attempts
¶ max vote attempts before stopped
-
mesh_addr_t
rc_addr
¶ root address specified by users via API esp_mesh_waive_root()
-
int
-
struct
mesh_event_find_network_t
¶ find a mesh network that this device can join
-
struct
mesh_event_root_switch_req_t
¶ Root switch request information.
Public Members
-
int
reason
¶ root switch reason, generally root switch is initialized by users via API esp_mesh_waive_root()
-
mesh_addr_t
rc_addr
¶ the address of root switch requester
-
int
-
struct
mesh_event_root_conflict_t
¶ Other powerful root address.
-
struct
mesh_event_routing_table_change_t
¶ Routing table change.
-
struct
mesh_event_scan_done_t
¶ Scan done event information.
Public Members
-
uint8_t
number
¶ the number of APs scanned
-
uint8_t
-
struct
mesh_event_network_state_t
¶ Network state information.
Public Members
-
bool
is_rootless
¶ whether current mesh network has a root
-
bool
-
struct
mesh_event_t
¶ Mesh event.
-
struct
mesh_opt_t
¶ Mesh option.
-
struct
mesh_data_t
¶ Mesh data for esp_mesh_send() and esp_mesh_recv()
Public Members
-
uint8_t *
data
¶ data
-
uint16_t
size
¶ data size
-
mesh_proto_t
proto
¶ data protocol
-
mesh_tos_t
tos
¶ data type of service
-
uint8_t *
-
struct
mesh_router_t
¶ Router configuration.
Public Members
-
uint8_t
ssid
[32]¶ SSID
-
uint8_t
ssid_len
¶ length of SSID
-
uint8_t
bssid
[6]¶ BSSID, if this value is specified, users should also specify “allow_router_switch”.
-
uint8_t
password
[64]¶ password
-
bool
allow_router_switch
¶ if the BSSID is specified and this value is also set, when the router of this specified BSSID fails to be found after “fail” (mesh_attempts_t) times, the whole network is allowed to switch to another router with the same SSID. The new router might also be on a different channel. The default value is false. There is a risk that if the password is different between the new switched router and the previous one, the mesh network could be established but the root will never connect to the new switched router.
-
uint8_t
-
struct
mesh_ap_cfg_t
¶ Mesh softAP configuration.
-
struct
mesh_cfg_t
¶ Mesh initialization configuration.
Public Members
-
uint8_t
channel
¶ channel, the mesh network on
-
bool
allow_channel_switch
¶ if this value is set, when “fail” (mesh_attempts_t) times is reached, device will change to a full channel scan for a network that could join. The default value is false.
-
mesh_event_cb_t
event_cb
¶ mesh event callback
-
mesh_addr_t
mesh_id
¶ mesh network identification
-
mesh_router_t
router
¶ router configuration
-
mesh_ap_cfg_t
mesh_ap
¶ mesh softAP configuration
-
const mesh_crypto_funcs_t *
crypto_funcs
¶ crypto functions
-
uint8_t
-
struct
mesh_vote_t
¶ Vote.
Public Members
-
float
percentage
¶ vote percentage threshold for approval of being a root
-
bool
is_rc_specified
¶ if true, rc_addr shall be specified (Unimplemented). if false, attempts value shall be specified to make network start root election.
-
mesh_rc_config_t
config
¶ vote address configuration
-
float
-
struct
mesh_tx_pending_t
¶ The number of packets pending in the queue waiting to be sent by the mesh stack.
-
struct
mesh_rx_pending_t
¶ The number of packets available in the queue waiting to be received by applications.
Macros¶
-
MESH_ROOT_LAYER
¶ root layer value
-
MESH_MTU
¶ max transmit unit(in bytes)
-
MESH_MPS
¶ max payload size(in bytes)
-
ESP_ERR_MESH_WIFI_NOT_START
¶ Mesh error code definition.
Wi-Fi isn’t started
-
ESP_ERR_MESH_NOT_INIT
¶ mesh isn’t initialized
-
ESP_ERR_MESH_NOT_CONFIG
¶ mesh isn’t configured
-
ESP_ERR_MESH_NOT_START
¶ mesh isn’t started
-
ESP_ERR_MESH_NOT_SUPPORT
¶ not supported yet
-
ESP_ERR_MESH_NOT_ALLOWED
¶ operation is not allowed
-
ESP_ERR_MESH_NO_MEMORY
¶ out of memory
-
ESP_ERR_MESH_ARGUMENT
¶ illegal argument
-
ESP_ERR_MESH_EXCEED_MTU
¶ packet size exceeds MTU
-
ESP_ERR_MESH_TIMEOUT
¶ timeout
-
ESP_ERR_MESH_DISCONNECTED
¶ disconnected with parent on station interface
-
ESP_ERR_MESH_QUEUE_FAIL
¶ queue fail
-
ESP_ERR_MESH_QUEUE_FULL
¶ queue full
-
ESP_ERR_MESH_NO_PARENT_FOUND
¶ no parent found to join the mesh network
-
ESP_ERR_MESH_NO_ROUTE_FOUND
¶ no route found to forward the packet
-
ESP_ERR_MESH_OPTION_NULL
¶ no option found
-
ESP_ERR_MESH_OPTION_UNKNOWN
¶ unknown option
-
ESP_ERR_MESH_XON_NO_WINDOW
¶ no window for software flow control on upstream
-
ESP_ERR_MESH_INTERFACE
¶ low-level Wi-Fi interface error
-
ESP_ERR_MESH_DISCARD_DUPLICATE
¶ discard the packet due to the duplicate sequence number
-
ESP_ERR_MESH_DISCARD
¶ discard the packet
-
ESP_ERR_MESH_VOTING
¶ vote in progress
-
MESH_DATA_ENC
¶ Flags bitmap for esp_mesh_send() and esp_mesh_recv()
data encrypted (Unimplemented)
-
MESH_DATA_P2P
¶ point-to-point delivery over the mesh network
-
MESH_DATA_FROMDS
¶ receive from external IP network
-
MESH_DATA_TODS
¶ identify this packet is target to external IP network
-
MESH_DATA_NONBLOCK
¶ esp_mesh_send() non-block
-
MESH_DATA_DROP
¶ in the situation of the root having been changed, identify this packet can be dropped by new root
-
MESH_DATA_GROUP
¶ identify this packet is target to a group address
-
MESH_OPT_SEND_GROUP
¶ Option definitions for esp_mesh_send() and esp_mesh_recv()
data transmission by group; used with esp_mesh_send() and shall have payload
-
MESH_OPT_RECV_DS_ADDR
¶ return a remote IP address; used with esp_mesh_send() and esp_mesh_recv()
-
MESH_ASSOC_FLAG_VOTE_IN_PROGRESS
¶ Flag of mesh networking IE.
vote in progress
-
MESH_ASSOC_FLAG_NETWORK_FREE
¶ no root in current network
-
MESH_ASSOC_FLAG_ROOTS_FOUND
¶ root conflict is found
-
MESH_ASSOC_FLAG_ROOT_FIXED
¶ fixed root
-
MESH_INIT_CONFIG_DEFAULT
()¶
Type Definitions¶
-
typedef system_event_sta_got_ip_t
mesh_event_root_got_ip_t
¶ IP settings from LwIP stack.
-
typedef mesh_addr_t
mesh_event_root_address_t
¶ Root address.
-
typedef system_event_sta_disconnected_t
mesh_event_disconnected_t
¶ Parent disconnected information.
-
typedef system_event_ap_staconnected_t
mesh_event_child_connected_t
¶ Child connected information.
-
typedef system_event_ap_stadisconnected_t
mesh_event_child_disconnected_t
¶ Child disconnected information.
-
typedef system_event_sta_connected_t
mesh_event_router_switch_t
¶ New router information.
-
typedef void (*
mesh_event_cb_t
)(mesh_event_t event)¶ Mesh event callback handler prototype definition.
- Parameters
event
: mesh_event_t
Enumerations¶
-
enum
mesh_event_id_t
¶ Enumerated list of mesh event id.
Values:
-
MESH_EVENT_STARTED
¶ mesh is started
-
MESH_EVENT_STOPPED
¶ mesh is stopped
-
MESH_EVENT_CHANNEL_SWITCH
¶ channel switch
-
MESH_EVENT_CHILD_CONNECTED
¶ a child is connected on softAP interface
-
MESH_EVENT_CHILD_DISCONNECTED
¶ a child is disconnected on softAP interface
-
MESH_EVENT_ROUTING_TABLE_ADD
¶ routing table is changed by adding newly joined children
-
MESH_EVENT_ROUTING_TABLE_REMOVE
¶ routing table is changed by removing leave children
-
MESH_EVENT_PARENT_CONNECTED
¶ parent is connected on station interface
-
MESH_EVENT_PARENT_DISCONNECTED
¶ parent is disconnected on station interface
-
MESH_EVENT_NO_PARENT_FOUND
¶ no parent found
-
MESH_EVENT_LAYER_CHANGE
¶ layer changes over the mesh network
-
MESH_EVENT_TODS_STATE
¶ state represents whether the root is able to access external IP network
-
MESH_EVENT_VOTE_STARTED
¶ the process of voting a new root is started either by children or by the root
-
MESH_EVENT_VOTE_STOPPED
¶ the process of voting a new root is stopped
-
MESH_EVENT_ROOT_ADDRESS
¶ the root address is obtained. It is posted by mesh stack automatically.
-
MESH_EVENT_ROOT_SWITCH_REQ
¶ root switch request sent from a new voted root candidate
-
MESH_EVENT_ROOT_SWITCH_ACK
¶ root switch acknowledgment responds the above request sent from current root
-
MESH_EVENT_ROOT_GOT_IP
¶ the root obtains the IP address. It is posted by LwIP stack automatically
-
MESH_EVENT_ROOT_LOST_IP
¶ the root loses the IP address. It is posted by LwIP stack automatically
-
MESH_EVENT_ROOT_ASKED_YIELD
¶ the root is asked yield by a more powerful existing root. If self organized is disabled and this device is specified to be a root by users, users should set a new parent for this device. if self organized is enabled, this device will find a new parent by itself, users could ignore this event.
-
MESH_EVENT_ROOT_FIXED
¶ when devices join a network, if the setting of Fixed Root for one device is different from that of its parent, the device will update the setting the same as its parent’s. Fixed Root Setting of each device is variable as that setting changes of the root.
-
MESH_EVENT_SCAN_DONE
¶ if self-organized networking is disabled, user can call esp_wifi_scan_start() to trigger this event, and add the corresponding scan done handler in this event.
-
MESH_EVENT_NETWORK_STATE
¶ network state, such as whether current mesh network has a root.
-
MESH_EVENT_STOP_RECONNECTION
¶ the root stops reconnecting to the router and non-root devices stop reconnecting to their parents.
-
MESH_EVENT_FIND_NETWORK
¶ when the channel field in mesh configuration is set to zero, mesh stack will perform a full channel scan to find a mesh network that can join, and return the channel value after finding it.
-
MESH_EVENT_ROUTER_SWITCH
¶ if users specify BSSID of the router in mesh configuration, when the root connects to another router with the same SSID, this event will be posted and the new router information is attached.
-
MESH_EVENT_MAX
¶
-
-
enum
mesh_type_t
¶ Device type.
Values:
-
MESH_IDLE
¶ hasn’t joined the mesh network yet
-
MESH_ROOT
¶ the only sink of the mesh network. Has the ability to access external IP network
-
MESH_NODE
¶ intermediate device. Has the ability to forward packets over the mesh network
-
MESH_LEAF
¶ has no forwarding ability
-
-
enum
mesh_proto_t
¶ Protocol of transmitted application data.
Values:
-
MESH_PROTO_BIN
¶ binary
-
MESH_PROTO_HTTP
¶ HTTP protocol
-
MESH_PROTO_JSON
¶ JSON format
-
MESH_PROTO_MQTT
¶ MQTT protocol
-
-
enum
mesh_tos_t
¶ For reliable transmission, mesh stack provides three type of services.
Values:
-
MESH_TOS_P2P
¶ provide P2P (point-to-point) retransmission on mesh stack by default
-
MESH_TOS_E2E
¶ provide E2E (end-to-end) retransmission on mesh stack (Unimplemented)
-
MESH_TOS_DEF
¶ no retransmission on mesh stack
-
-
enum
mesh_vote_reason_t
¶ Vote reason.
Values:
-
MESH_VOTE_REASON_ROOT_INITIATED
= 1¶ vote is initiated by the root
-
MESH_VOTE_REASON_CHILD_INITIATED
¶ vote is initiated by children
-
-
enum
mesh_disconnect_reason_t
¶ Mesh disconnect reason code.
Values:
-
MESH_REASON_CYCLIC
= 100¶ cyclic is detected
-
MESH_REASON_PARENT_IDLE
¶ parent is idle
-
MESH_REASON_LEAF
¶ the connected device is changed to a leaf
-
MESH_REASON_DIFF_ID
¶ in different mesh ID
-
MESH_REASON_ROOTS
¶ root conflict is detected
-
MESH_REASON_PARENT_STOPPED
¶ parent has stopped the mesh
-
MESH_REASON_SCAN_FAIL
¶ scan fail
-
MESH_REASON_IE_UNKNOWN
¶ unknown IE
-
MESH_REASON_WAIVE_ROOT
¶ waive root
-
MESH_REASON_PARENT_WORSE
¶ parent with very poor RSSI
-
MESH_REASON_EMPTY_PASSWORD
¶ use an empty password to connect to an encrypted parent
-
MESH_REASON_PARENT_UNENCRYPTED
¶ connect to an unencrypted parent/router
-