Sigma-delta Modulation¶
Introduction¶
ESP32 has a second-order sigma-delta modulation module. This driver configures the channels of the sigma-delta module.
Functionality Overview¶
There are 8 independent sigma-delta modulation channels identified with sigmadelta_channel_t
. Each channel is capable to output the binary, hardware generated signal with the sigma-delta modulation.
Selected channel should be set up by providing configuration parameters in sigmadelta_config_t
and then applying this configuration with sigmadelta_config()
.
Another option is to call individual functions, that will configure all required parameters one by one:
Prescaler of the sigma-delta generator -
sigmadelta_set_prescale()
Duty of the output signal -
sigmadelta_set_duty()
GPIO pin to output modulated signal -
sigmadelta_set_pin()
The range of the ‘duty’ input parameter of sigmadelta_set_duty()
is from -128 to 127 (eight bit signed integer). If zero value is set, then the output signal’s duty will be about 50%, see description of sigmadelta_set_duty()
.
Convert to analog signal (Optional)¶
Typically, if the sigma-delta signal is connected to an LED, you don’t have to add any filter between them (because our eyes are a low pass filter naturally). However, if you want to check the real voltage or watch the analog waveform, you need to design an analog low pass filter. Also, it is recommended to use an active filter instead of a passive filter to gain better isolation and not lose too much voltage.
For example, you can take the following Sallen-Key topology Low Pass Filter as a reference.
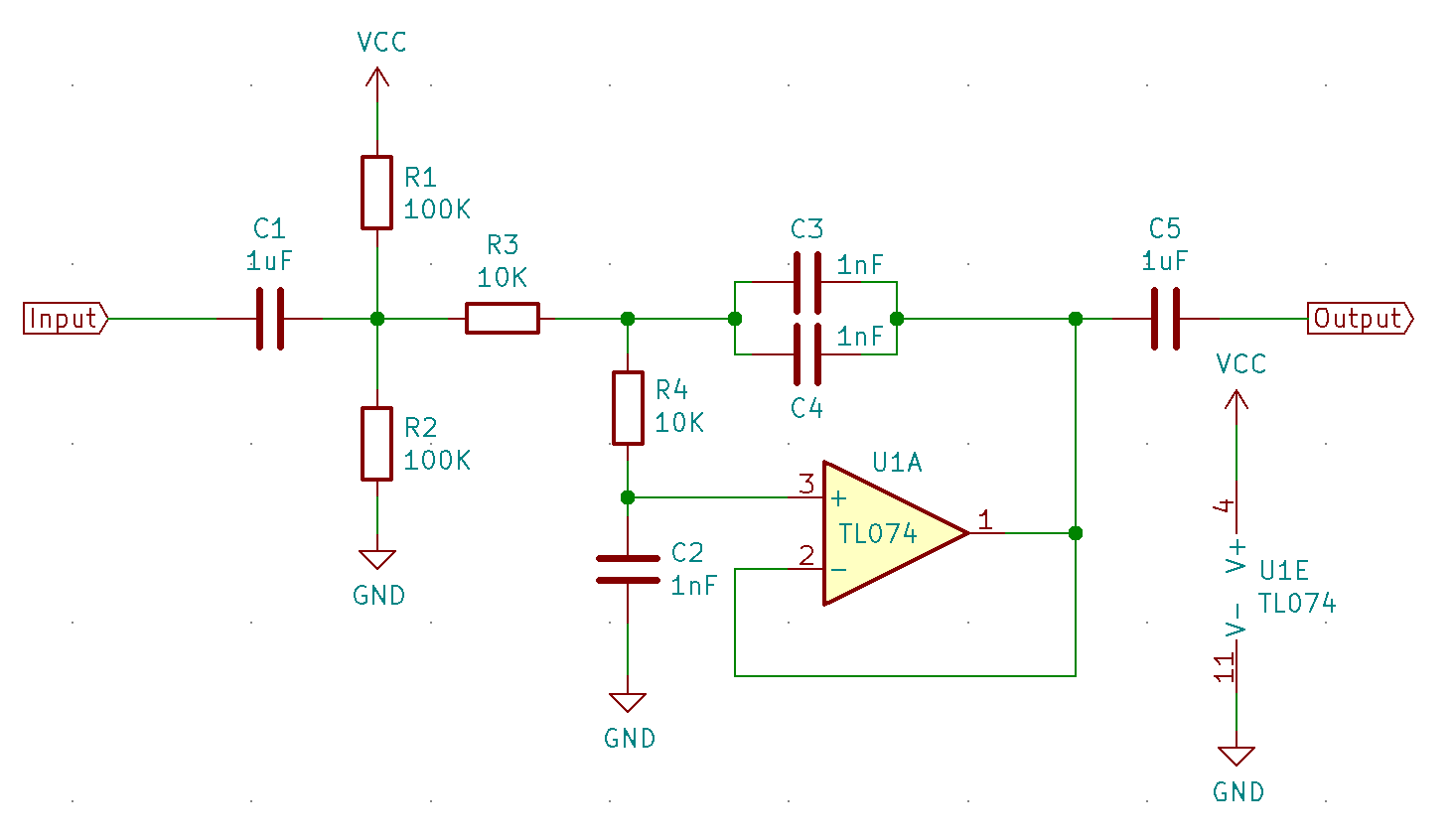
Sallen-Key Low Pass Filter¶
Application Example¶
Sigma-delta Modulation example: peripherals/sigmadelta.
API Reference¶
Header File¶
Functions¶
-
esp_err_t
sigmadelta_config
(const sigmadelta_config_t *config)¶ Configure Sigma-delta channel.
- Return
ESP_OK Success
ESP_ERR_INVALID_STATE sigmadelta driver already initialized
ESP_ERR_INVALID_ARG Parameter error
- Parameters
config
: Pointer of Sigma-delta channel configuration struct
-
esp_err_t
sigmadelta_set_duty
(sigmadelta_channel_t channel, int8_t duty)¶ Set Sigma-delta channel duty.
This function is used to set Sigma-delta channel duty, If you add a capacitor between the output pin and ground, the average output voltage will be Vdc = VDDIO / 256 * duty + VDDIO/2, where VDDIO is the power supply voltage.
- Return
ESP_OK Success
ESP_ERR_INVALID_STATE sigmadelta driver has not been initialized
ESP_ERR_INVALID_ARG Parameter error
- Parameters
channel
: Sigma-delta channel numberduty
: Sigma-delta duty of one channel, the value ranges from -128 to 127, recommended range is -90 ~ 90. The waveform is more like a random one in this range.
-
esp_err_t
sigmadelta_set_prescale
(sigmadelta_channel_t channel, uint8_t prescale)¶ Set Sigma-delta channel’s clock pre-scale value. The source clock is APP_CLK, 80MHz. The clock frequency of the sigma-delta channel is APP_CLK / pre_scale.
- Return
ESP_OK Success
ESP_ERR_INVALID_STATE sigmadelta driver has not been initialized
ESP_ERR_INVALID_ARG Parameter error
- Parameters
channel
: Sigma-delta channel numberprescale
: The divider of source clock, ranges from 0 to 255
-
esp_err_t
sigmadelta_set_pin
(sigmadelta_channel_t channel, gpio_num_t gpio_num)¶ Set Sigma-delta signal output pin.
- Return
ESP_OK Success
ESP_ERR_INVALID_STATE sigmadelta driver has not been initialized
ESP_ERR_INVALID_ARG Parameter error
- Parameters
channel
: Sigma-delta channel numbergpio_num
: GPIO number of output pin.
Header File¶
Structures¶
-
struct
sigmadelta_config_t
¶ Sigma-delta configure struct.
Public Members
-
sigmadelta_channel_t
channel
¶ Sigma-delta channel number
-
int8_t
sigmadelta_duty
¶ Sigma-delta duty, duty ranges from -128 to 127.
-
uint8_t
sigmadelta_prescale
¶ Sigma-delta prescale, prescale ranges from 0 to 255.
-
uint8_t
sigmadelta_gpio
¶ Sigma-delta output io number, refer to gpio.h for more details.
-
sigmadelta_channel_t
Enumerations¶
-
enum
sigmadelta_port_t
¶ SIGMADELTA port number, the max port number is (SIGMADELTA_NUM_MAX -1).
Values:
-
SIGMADELTA_PORT_0
¶ SIGMADELTA port 0
-
SIGMADELTA_PORT_MAX
¶ SIGMADELTA port max
-
-
enum
sigmadelta_channel_t
¶ Sigma-delta channel list.
Values:
-
SIGMADELTA_CHANNEL_0
¶ Sigma-delta channel 0
-
SIGMADELTA_CHANNEL_1
¶ Sigma-delta channel 1
-
SIGMADELTA_CHANNEL_2
¶ Sigma-delta channel 2
-
SIGMADELTA_CHANNEL_3
¶ Sigma-delta channel 3
-
SIGMADELTA_CHANNEL_4
¶ Sigma-delta channel 4
-
SIGMADELTA_CHANNEL_5
¶ Sigma-delta channel 5
-
SIGMADELTA_CHANNEL_6
¶ Sigma-delta channel 6
-
SIGMADELTA_CHANNEL_7
¶ Sigma-delta channel 7
-
SIGMADELTA_CHANNEL_MAX
¶ Sigma-delta channel max
-