Sensor Hub
Sensor Hub is a sensor management component that can realize hardware abstraction, device management and data distribution for sensor devices. When developing applications based on Sensor Hub, users do not have to deal with complex sensor implementations, but only need to make simple selections for sensor operation, acquisition interval, range, etc. and then register callback functions to the event messages of your interests. By doing so, users can receive notifications when sensor states are switched or when data is collected.
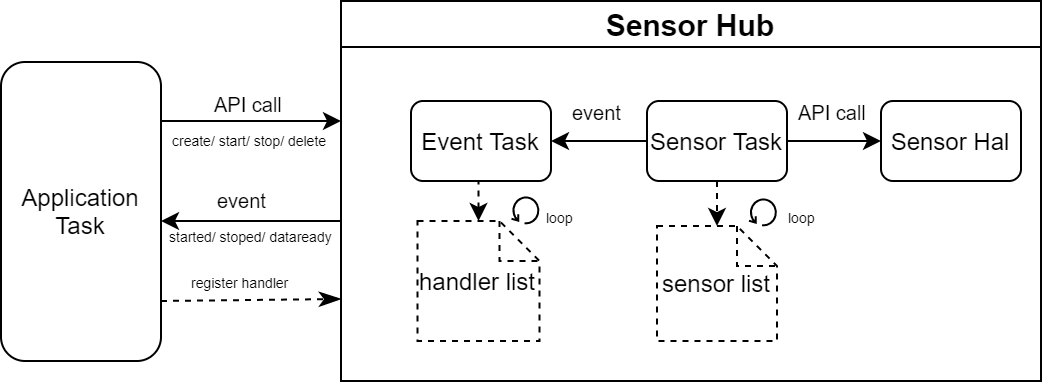
Sensor Hub Programming Model
Sensor Hub supports loading sensor drivers as components. Users only need to input the name of the sensor registered with the Sensor Hub to directly access the corresponding sensor driver. Additionally, as this component enables centralized management of sensors, it not only simplifies operations but also improves operational efficiency. It can serve as a foundational component for sensor applications and can be applied to various intelligent scenarios such as environmental sensing, motion sensing, health management, and more.

Sensor Hub Driver
Instructions
sensor_hub
uses the linker script generation mechanism to register sensor drivers into specific target file sections. For application developers, there is no need to focus on the specific implementation of the sensor drivers; simply adding the corresponding sensor component will load the respective driver.
Driver developer:
Taking the ShT3X
temperature and humidity sensor as an example, driver developers need to populate the humiture_impl_t
structure with operations related to the sensor and create a sensor detection function. sensor_hub
provides a sensor registration interface: SENSOR_HUB_DETECT_FN
, where driver developers can directly insert the corresponding functions into the registration interface.
static humiture_impl_t sht3x_impl = {
.init = humiture_sht3x_init,
.deinit = humiture_sht3x_deinit,
.test = humiture_sht3x_test,
.acquire_humidity = humiture_sht3x_acquire_humidity,
.acquire_temperature = humiture_sht3x_acquire_temperature,
};
SENSOR_HUB_DETECT_FN(HUMITURE_ID, sht3x, &sht3x_impl);
At the same time, add the interface dependency in the component’s CMakeLists.txt
:
target_link_libraries(${COMPONENT_LIB} INTERFACE "-u humiture_sht3x_init")
Application developer
Add
sensor_hub
and the required sensor components in the project’sidf_component.yml
.Create a sensor instance: Use
iot_sensor_create()
to create a sensor instance. The parameters include the sensor name, sensor configuration options, and a pointer to the sensor handle. The sensor name is used to locate and load the driver registered in thesensor_hub
. If the sensor supports configurable addresses, multiple instances of the same sensor name can be created. In the configuration options,bus
specifies the bus to which the sensor is mounted;addr
specifies the address corresponding to the sensor;type
specifies the type of the sensor ;mode
specifies the operating mode of the sensor; andmin_delay
specifies the sensor’s data collection interval. Other options are optional. Upon successful creation, the sensor handle is obtained.Register callback functions for sensor events: when a sensor event occurs, the callback functions will be called in sequence. There are two ways to register a callback function, and the instance handler of the event callback function will be returned after the registration succeed:
Use
iot_sensor_handler_register()
to register a callback function with the sensor handlerUse
iot_sensor_handler_register_with_type()
to register a callback function with the sensor type
Start a sensor: use
iot_sensor_start()
to start a specific sensor. After started, it will trigger aSENSOR_STARTED
event, then it will collect the sensor data continuously with a set of period and triggerSENSOR_XXXX_DATA_READY
event. The event callback function can obtain the specific data of each event via theevent_data
parameter;Stop a sensor: use
iot_sensor_stop()
to stop a specified sensor temporarily. After stopped, the sensor will send out aSENSOR_STOPED
event and then stop the data collecting work. If the driver of this sensor supports power management, the sensor will be set to sleep mode in this stage;Unregister callback functions for sensor events: the user program can unregister an event at any time using the instance handler of this event callback function, and this callback function will not be called again when this event occurs afterwards. There are also two ways to do so:
Use
iot_sensor_handler_unregister()
to unregister the callback function with the sensor handlerUse
iot_sensor_handler_unregister_with_type()
to unregister the callback function with the sensor type
Delete sensors: use
iot_sensor_delete()
to delete the corresponding sensor to release the allocated memory and other resources.
Examples
Sensor control LED example: sensors/sensor_control_led.
Sensor hub monitor example: sensors/sensor_hub_monitor.
API Reference
Header File
Structures
-
struct sensor_data_t
sensor data type
Public Members
-
int64_t timestamp
timestamp
-
const char *sensor_name
sensor name
-
sensor_type_t sensor_type
sensor type
-
uint8_t sensor_addr
sensor addr
-
int32_t event_id
reserved for future use
-
uint32_t min_delay
minimum delay between two events, unit: ms
-
axis3_t acce
Accelerometer. unit: G
-
axis3_t gyro
Gyroscope. unit: dps
-
axis3_t mag
Magnetometer. unit: Gauss
-
float temperature
Temperature. unit: dCelsius
-
float humidity
Relative humidity. unit: percentage
-
float baro
Pressure. unit: pascal (Pa)
-
float light
Light. unit: lux
-
rgbw_t rgbw
Color. unit: lux
-
uv_t uv
ultraviole unit: lux
-
float proximity
Distance. unit: centimeters
-
float hr
Heat rate. unit: HZ
-
float tvoc
TVOC. unit: permillage
-
float noise
Noise Loudness. unit: HZ
-
float step
Step sensor. unit: 1
-
float force
Force sensor. unit: mN
-
float current
Current sensor unit: mA
-
float voltage
Voltage sensor unit: mV
-
float data[4]
for general use
-
int64_t timestamp
-
struct sensor_data_group_t
sensor data group type
Public Members
-
uint8_t number
effective data number
-
sensor_data_t sensor_data[SENSOR_DATA_GROUP_MAX_NUM]
data buffer
-
uint8_t number
Macros
-
SENSOR_EVENT_ANY_ID
register handler for any event id
Type Definitions
-
typedef void *sensor_device_impl_t
-
typedef void *sensor_driver_handle_t
hal level sensor driver handle
-
typedef void *bus_handle_t
i2c/spi bus handle
Enumerations
-
enum sensor_type_t
sensor type
Values:
-
enumerator NULL_ID
NULL
-
enumerator HUMITURE_ID
humidity or temperature sensor
-
enumerator IMU_ID
gyro or acc sensor
-
enumerator LIGHT_SENSOR_ID
light illumination or uv or color sensor
-
enumerator SENSOR_TYPE_MAX
max sensor type
-
enumerator NULL_ID
-
enum sensor_command_t
sensor operate command
Values:
-
enumerator COMMAND_SET_MODE
set measure mode
-
enumerator COMMAND_SET_RANGE
set measure range
-
enumerator COMMAND_SET_ODR
set output rate
-
enumerator COMMAND_SET_POWER
set power mode
-
enumerator COMMAND_SELF_TEST
sensor self test
-
enumerator COMMAND_MAX
max sensor command
-
enumerator COMMAND_SET_MODE
-
enum sensor_power_mode_t
sensor power mode
Values:
-
enumerator POWER_MODE_WAKEUP
wakeup from sleep
-
enumerator POWER_MODE_SLEEP
set to sleep
-
enumerator POWER_MAX
max sensor power mode
-
enumerator POWER_MODE_WAKEUP
-
enum sensor_mode_t
sensor acquire mode
Values:
-
enumerator MODE_DEFAULT
default work mode
-
enumerator MODE_POLLING
polling acquire with a interval time
-
enumerator MODE_INTERRUPT
interrupt mode, acquire data when interrupt comes
-
enumerator MODE_MAX
max sensor mode
-
enumerator MODE_DEFAULT
-
enum sensor_range_t
sensor acquire range
Values:
-
enumerator RANGE_DEFAULT
default range
-
enumerator RANGE_MIN
minimum range for high-speed or high-precision
-
enumerator RANGE_MEDIUM
medium range for general use
-
enumerator RANGE_MAX
maximum range for full scale
-
enumerator RANGE_DEFAULT
-
enum sensor_event_id_t
sensor general events
Values:
-
enumerator SENSOR_STARTED
sensor started, data acquire will be started
-
enumerator SENSOR_STOPED
sensor stopped, data acquire will be stopped
-
enumerator SENSOR_EVENT_COMMON_END
max common events id
-
enumerator SENSOR_STARTED
-
enum sensor_data_event_id_t
sensor data ready events
Values:
-
enumerator SENSOR_ACCE_DATA_READY
Accelerometer data ready
-
enumerator SENSOR_GYRO_DATA_READY
Gyroscope data ready
-
enumerator SENSOR_MAG_DATA_READY
Magnetometer data ready
-
enumerator SENSOR_TEMP_DATA_READY
Temperature data ready
-
enumerator SENSOR_HUMI_DATA_READY
Relative humidity data ready
-
enumerator SENSOR_BARO_DATA_READY
Pressure data ready
-
enumerator SENSOR_LIGHT_DATA_READY
Light data ready
-
enumerator SENSOR_RGBW_DATA_READY
Color data ready
-
enumerator SENSOR_UV_DATA_READY
ultraviolet data ready
-
enumerator SENSOR_PROXI_DATA_READY
Distance data ready
-
enumerator SENSOR_HR_DATA_READY
Heat rate data ready
-
enumerator SENSOR_TVOC_DATA_READY
TVOC data ready
-
enumerator SENSOR_NOISE_DATA_READY
Noise Loudness data ready
-
enumerator SENSOR_STEP_DATA_READY
Step data ready
-
enumerator SENSOR_FORCE_DATA_READY
Force data ready
-
enumerator SENSOR_CURRENT_DATA_READY
Current data ready
-
enumerator SENSOR_VOLTAGE_DATA_READY
Voltage data ready
-
enumerator SENSOR_EVENT_ID_END
max common events id
-
enumerator SENSOR_ACCE_DATA_READY
Header File
Functions
-
esp_err_t iot_sensor_create(const char *sensor_name, const sensor_config_t *config, sensor_handle_t *p_sensor_handle)
Create a sensor instance with specified name and desired configurations.
- Parameters
sensor_name – sensor’s name. Sensor information will be registered by ESP_SENSOR_DETECT_FN
config – sensor’s configurations detailed in sensor_config_t
p_sensor_handle – return sensor handle if succeed, NULL if failed.
- Returns
esp_err_t
ESP_OK Success
ESP_FAIL Fail
-
esp_err_t iot_sensor_start(sensor_handle_t sensor_handle)
start sensor acquisition, post data ready events when data acquired. if start succeed, sensor will start to acquire data with desired mode and post events in min_delay(ms) intervals SENSOR_STARTED event will be posted.
- Parameters
sensor_handle – sensor handle for operation
- Returns
esp_err_t
ESP_OK Success
ESP_FAIL Fail
-
esp_err_t iot_sensor_stop(sensor_handle_t sensor_handle)
stop sensor acquisition, and stop post data events. if stop succeed, SENSOR_STOPED event will be posted.
- Parameters
sensor_handle – sensor handle for operation
- Returns
esp_err_t
ESP_OK Success
ESP_FAIL Fail
-
esp_err_t iot_sensor_delete(sensor_handle_t p_sensor_handle)
delete and release the sensor resource.
- Parameters
p_sensor_handle – sensor handle, will set to NULL if delete succeed.
- Returns
esp_err_t
ESP_OK Success
ESP_FAIL Fail
-
int iot_sensor_scan()
Scan for valid sensors attached on bus.
Scan for valid sensors registered in the system
- Parameters
bus – bus handle
buf – Pointer to a buffer to save sensors’ information, if NULL no information will be saved.
num – Maximum number of sensor information to save, invalid if buf set to NULL, latter sensors will be discarded if num less-than the total number found on the bus.
- Returns
uint8_t total number of valid sensors found on the bus
- Returns
int number of valid sensors
-
esp_err_t iot_sensor_handler_register(sensor_handle_t sensor_handle, sensor_event_handler_t handler, sensor_event_handler_instance_t *context)
Register a event handler to a sensor’s event with sensor_handle.
- Parameters
sensor_handle – sensor handle for operation
handler – the handler function which gets called when the sensor’s any event is dispatched
context – An event handler instance object related to the registered event handler and data, can be NULL. This needs to be kept if the specific callback instance should be unregistered before deleting the whole event loop. Registering the same event handler multiple times is possible and yields distinct instance objects. The data can be the same for all registrations. If no unregistration is needed but the handler should be deleted when the event loop is deleted, instance can be NULL.
- Returns
esp_err_t
ESP_OK Success
ESP_FAIL Fail
-
esp_err_t iot_sensor_handler_unregister(sensor_handle_t sensor_handle, sensor_event_handler_instance_t context)
Unregister a event handler from a sensor’s event.
- Parameters
sensor_handle – sensor handle for operation
context – the instance object of the registration to be unregistered
- Returns
esp_err_t
ESP_OK Success
ESP_FAIL Fail
-
esp_err_t iot_sensor_handler_register_with_type(sensor_type_t sensor_type, int32_t event_id, sensor_event_handler_t handler, sensor_event_handler_instance_t *context)
Register a event handler with sensor_type instead of sensor_handle. the api only care about the event type, don’t care who post it.
- Parameters
sensor_type – sensor type declared in sensor_type_t.
event_id – sensor event declared in sensor_event_id_t and sensor_data_event_id_t
handler – the handler function which gets called when the event is dispatched
context – An event handler instance object related to the registered event handler and data, can be NULL. This needs to be kept if the specific callback instance should be unregistered before deleting the whole event loop. Registering the same event handler multiple times is possible and yields distinct instance objects. The data can be the same for all registrations. If no unregistration is needed but the handler should be deleted when the event loop is deleted, instance can be NULL.
- Returns
esp_err_t
ESP_OK Success
ESP_FAIL Fail
-
esp_err_t iot_sensor_handler_unregister_with_type(sensor_type_t sensor_type, int32_t event_id, sensor_event_handler_instance_t context)
Unregister a event handler from a event. the api only care about the event type, don’t care who post it.
- Parameters
sensor_type – sensor type declared in sensor_type_t.
event_id – sensor event declared in sensor_event_id_t and sensor_data_event_id_t
context – the instance object of the registration to be unregistered
- Returns
esp_err_t
ESP_OK Success
ESP_FAIL Fail
Structures
-
struct sensor_info_t
sensor information, written by the driver developer.
-
struct sensor_config_t
sensor initialization parameter
Public Members
-
bus_handle_t bus
i2c/spi bus handle
-
uint8_t addr
set addr of sensor
-
sensor_type_t type
sensor type
-
sensor_mode_t mode
set acquire mode detiled in sensor_mode_t
-
sensor_range_t range
set measuring range
-
uint32_t min_delay
set minimum acquisition interval
-
int intr_pin
set interrupt pin
-
int intr_type
set interrupt type
-
bus_handle_t bus
-
struct sensor_hub_detect_fn_t
Detection function interface for sensors.
Public Members
-
void *(*fn)(sensor_info_t *sensor_info)
Function pointer for sensor detection.
This function detects a sensor based on the provided sensor information.
- Param sensor_info
Pointer to the sensor information structure
- Return
void* Pointer to the detected sensor instance, or NULL if detection failed
-
void *(*fn)(sensor_info_t *sensor_info)
Macros
-
SENSOR_HUB_DETECT_FN(type_id, name_id, impl)
Defines a sensor detection function to be executed automatically in the application layer.
This macro defines a function and its corresponding metadata to facilitate automatic sensor detection and initialization. The function must return the implementation (
impl
) on success; otherwise, an error is logged, and the automatic detection process in the application layer will be aborted.To prevent the linker from optimizing out the sensor driver, the driver must include at least one undefined symbol that is explicitly referenced during the linking phase. This ensures that the linker retains the driver, even if no other files directly depend on its symbols.
For example, in the sensor driver’s
CMakeLists.txt
, include the following to force the linker to keep the required symbol:target_link_libraries(${COMPONENT_LIB} INTERFACE “-u <symbol_name>”)
Replace
<symbol_name>
with an appropriate symbol, such assht3x_init
, defined in the driver.- Parameters
type_id – The sensor type identifier.
name_id – The unique name identifier for the sensor.
impl – The implementation returned on successful detection.
Type Definitions
-
typedef void *sensor_handle_t
sensor handle
-
typedef void *sensor_event_handler_instance_t
sensor event handler handle
-
typedef void (*sensor_event_handler_t)(void *event_handler_arg, sensor_event_base_t event_base, int32_t event_id, void *event_data)
function called when an event is posted to the queue