Touch Element
Overview
The Touch Element Library is a highly abstracted element library designed on the basis of the touch sensor driver. The library provides a unified and user-friendly software interface to quickly build capacitive touch sensor applications. For more information about the touch sensor driver API, see Touch Sensor.
Architecture
The Touch Element library configures touch sensor peripherals via the touch sensor driver. However, some necessary hardware parameters should be passed to touch_element_install()
and will be configured automatically only after calling touch_element_start()
. This sequential order is essential because configuring these parameters has a significant impact on the run-time system. Therefore, they must be configured after calling the start function to ensure the system functions properly.
These parameters include touch channel threshold, driver-level of waterproof shield sensor, etc. The Touch Element library sets the touch sensor interrupt and the esp_timer routine up, and the hardware information of the touch sensor (channel state, channel number) will be obtained in the touch sensor interrupt service routine. When the specified channel event occurs, the hardware information is passed to the esp_timer callback routine, which then dispatches the touch sensor channel information to the touch elements (such as button, slider, etc.). The library then runs a specified algorithm to update the touch element's state or calculate its position and dispatches the result accordingly.
So when using the Touch Element library, you are relieved from the implementation details of the touch sensor peripheral. The library handles most of the hardware information and passes the more meaningful messages to the event handler routine.
The workflow of the Touch Element library is illustrated in the picture below.
Touch Element architecture
The features in relation to the Touch Element library in ESP32-S2 are given in the table below.
Features |
ESP32S2 |
---|---|
Touch Element waterproof |
✔ |
Touch Element button |
✔ |
Touch Element slider |
✔ |
Touch Element matrix button |
✔ |
Peripheral
ESP32-S2 integrates one touch sensor peripheral with several physical channels.
14 physical capacitive touch channels
Timer or software FSM trigger mode
Up to 5 kinds of interrupt (Upper threshold and lower threshold interrupt, measure one channel finish and measure all channels finish interrupt, measurement timeout interrupt)
Sleep mode wakeup source
Hardware internal de-noise
Hardware filter
Hardware waterproof sensor
Hardware proximity sensor
The channels are located as follows:
Channel |
ESP32-S2 |
---|---|
Channel 0 |
GPIO 0 (reserved) |
Channel 1 |
GPIO 1 |
Channel 2 |
GPIO 2 |
Channel 3 |
GPIO 3 |
Channel 4 |
GPIO 4 |
Channel 5 |
GPIO 5 |
Channel 6 |
GPIO 6 |
Channel 7 |
GPIO 7 |
Channel 8 |
GPIO 8 |
Channel 9 |
GPIO 9 |
Channel 10 |
GPIO 10 |
Channel 11 |
GPIO 11 |
Channel 12 |
GPIO 12 |
Channel 13 |
GPIO 13 |
Channel 14 |
GPIO 14 |
The channels are located as follows:
Channel |
ESP32-S2 |
---|---|
Channel 0 |
GPIO 0 (effective) |
Channel 1 |
GPIO 1 |
Channel 2 |
GPIO 2 |
Channel 3 |
GPIO 3 |
Channel 4 |
GPIO 4 |
Channel 5 |
GPIO 5 |
Channel 6 |
GPIO 6 |
Channel 7 |
GPIO 7 |
Channel 8 |
GPIO 8 |
Channel 9 |
GPIO 9 |
Terminology
The terms used in relation to the Touch Element library are given below.
Term |
Definition |
---|---|
Touch sensor |
Touch sensor peripheral inside the chip |
Touch channel |
Touch sensor channels inside the touch sensor peripheral |
Touch pad |
Off-chip physical solder pad, generally inside the PCB |
De-noise channel |
Internal de-noise channel, which is always Channel 0 and is reserved |
Shield sensor |
One of the waterproof sensors for detecting droplets in small areas and compensating for the influence of water drops on measurements |
Guard sensor |
One of the waterproof sensors for detecting extensive wading and to temporarily disable the touch sensor |
Shield channel |
The channel that waterproof shield sensor connected to, which is always Channel 14 |
Guard channel |
The channel that waterproof guard sensor connected to |
Shield pad |
Off-chip physical solder pad, generally is grids, and is connected to shield the sensor |
Guard pad |
Off-chip physical solder pad, usually a ring, and is connected to the guard sensor |
Touch sensor application system components
Touch Sensor Signal
Each touch sensor is able to provide the following types of signals:
Raw: The Raw signal is the unfiltered signal from the touch sensor.
Smooth: The Smooth signal is a filtered version of the Raw signal via an internal hardware filter.
Benchmark: The Benchmark signal is also a filtered signal that filters out extremely low-frequency noise.
All of these signals can be obtained using touch sensor driver API.
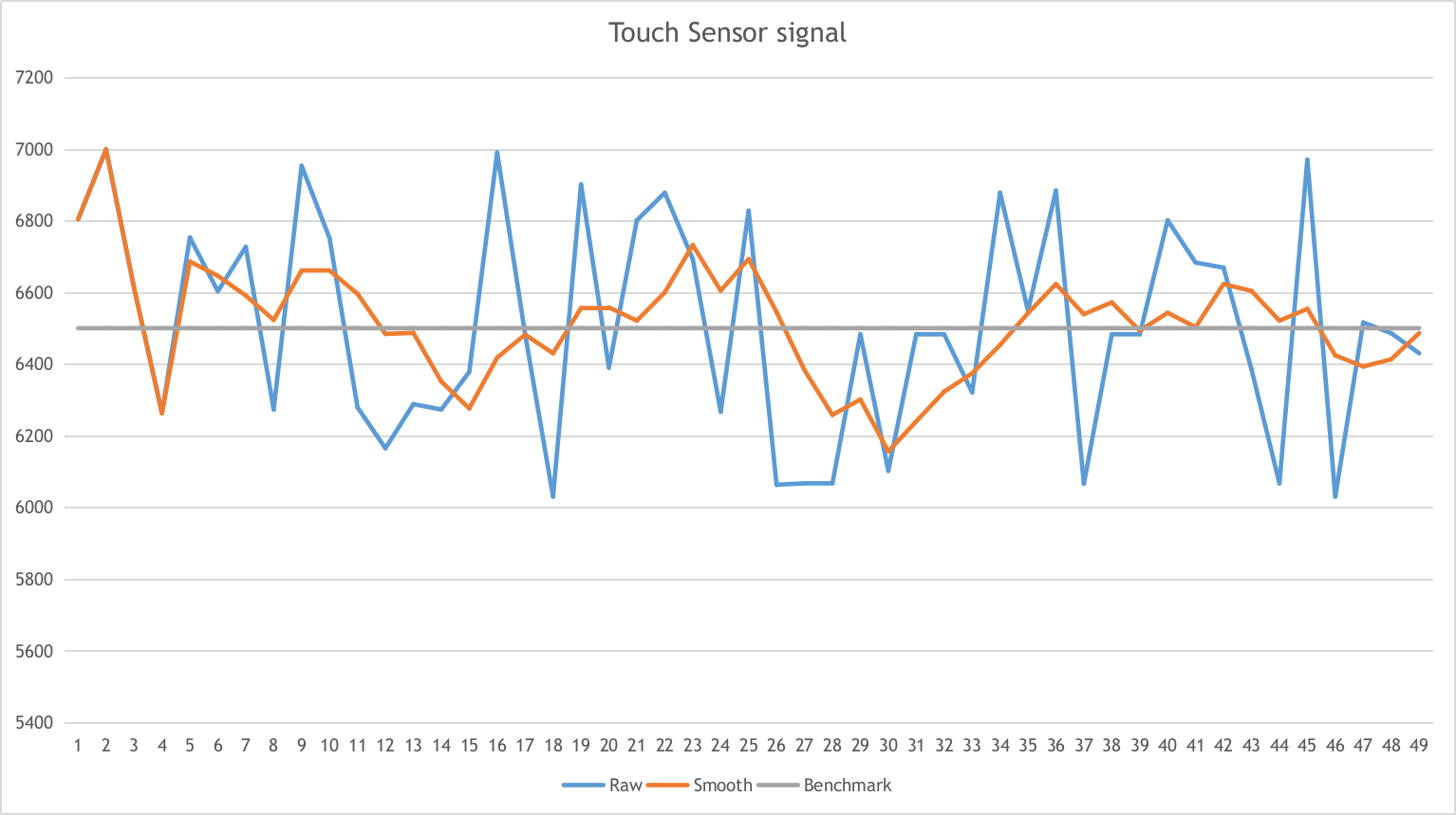
Touch sensor signals
Touch Sensor Signal Threshold
The Touch Sensor Threshold value is a configurable threshold value used to determine when a touch sensor is touched or not. When the difference between the Smooth signal and the Benchmark signal becomes greater than the threshold value (i.e., (smooth - benchmark) > threshold
), the touch channel's state will be changed and a touch interrupt will be triggered simultaneously.
Touch sensor signal threshold
Sensitivity
Important performance parameter of the touch sensor, the larger it is, the better touch the sensor performs. It could be calculated by the format below:
Waterproof
Waterproof is the hardware feature of a touch sensor which has a guard sensor and shield sensor (always connect to Channel 14) that has the ability to resist a degree of influence of water drop and detect the water stream.
Touch Slider
The touch slider consumes several channels (at least three channels) of the touch sensor, the more channels consumed, the higher resolution and accuracy position it performs. The touch slider looks like as the picture below:
Touch slider
Touch Matrix
The touch matrix button consumes several channels (at least 2 + 2 = 4 channels), and it gives a solution to use fewer channels and get more buttons. ESP32-S2 supports up to 49 buttons. The touch matrix button looks like as the picture below:
Touch matrix
Touch Element Library Usage
Using this library should follow the initialization flow below:
To initialize the Touch Element library by calling
touch_element_install()
.To initialize touch elements (button/slider etc) by calling
touch_xxxx_install()
.To create a new element instance by calling
touch_xxxx_create()
.To subscribe events by calling
touch_xxxx_subscribe_event()
.To choose a dispatch method by calling
touch_xxxx_set_dispatch_method()
that tells the library how to notify you while the subscribed event occurs.If dispatch by callback, call
touch_xxxx_set_callback()
to set the event handler function.To start the Touch Element library by calling
touch_element_start()
.If dispatch by callback, the callback will be called by the driver core when an event happens, no need to do anything; If dispatch by event task, create an event task and call
touch_element_message_receive()
to obtain messages in a loop.(Optional) If you want to suspend the Touch Element run-time system or for some reason that could not obtain the touch element message,
touch_element_stop()
should be called to suspend the Touch Element system and then resume it by callingtouch_element_start()
again.
In code, the flow above may look like as follows:
static touch_xxx_handle_t element_handle; //Declare a touch element handle
//Define the subscribed event handler
void event_handler(touch_xxx_handle_t out_handle, touch_xxx_message_t out_message, void *arg)
{
//Event handler logic
}
void app_main()
{
//Using the default initializer to config Touch Element library
touch_elem_global_config_t global_config = TOUCH_ELEM_GLOBAL_DEFAULT_CONFIG();
touch_element_install(&global_config);
//Using the default initializer to config Touch elements
touch_xxx_global_config_t elem_global_config = TOUCH_XXXX_GLOBAL_DEFAULT_CONFIG();
touch_xxx_install(&elem_global_config);
//Create a new instance
touch_xxx_config_t element_config = {
...
...
};
touch_xxx_create(&element_config, &element_handle);
//Subscribe the specified events by using the event mask
touch_xxx_subscribe_event(element_handle, TOUCH_ELEM_EVENT_ON_PRESS | TOUCH_ELEM_EVENT_ON_RELEASE, NULL);
//Choose CALLBACK as the dispatch method
touch_xxx_set_dispatch_method(element_handle, TOUCH_ELEM_DISP_CALLBACK);
//Register the callback routine
touch_xxx_set_callback(element_handle, event_handler);
//Start Touch Element library processing
touch_element_start();
}
Initialization
To initialize the Touch Element library, you have to configure the touch sensor peripheral and Touch Element library by calling
touch_element_install()
withtouch_elem_global_config_t
, the default initializer is available inTOUCH_ELEM_GLOBAL_DEFAULT_CONFIG()
and this default configuration is suitable for the most general application scene, and it is suggested not to change the default configuration before fully understanding Touch Sensor peripheral because some changes might bring several impacts to the system.To initialize the specified element, all the elements will not work before its constructor
touch_xxxx_install()
is called so as to save memory, so you have to call the constructor of each used touch element respectively, to set up the specified element.
Touch Element Instance Startup
To create a new touch element instance, call
touch_xxxx_create()
, select a channel, and provide its Sensitivity value for the new element instance.To subscribe to events, call
touch_xxxx_subscribe_event()
. The Touch Element library offers several events, and the event mask is available in components/touch_element/include/touch_element/touch_element.h. You can use these event masks to subscribe to specific events individually or combine them to subscribe to multiple events.To configure the dispatch method, use
touch_xxxx_set_dispatch_method()
. The Touch Element library provides two dispatch methods:TOUCH_ELEM_DISP_EVENT
andTOUCH_ELEM_DISP_CALLBACK
. These methods allow you to obtain the touch element message and handle it using different approaches.
Events Processing
If TOUCH_ELEM_DISP_EVENT
dispatch method is configured, you need to start up an event handler task to obtain the touch element message, all the elements' raw message could be obtained by calling touch_element_message_receive()
, then extract the element-class-specific message by calling the corresponding message decoder with touch_xxxx_get_message()
to get the touch element's extracted message; If TOUCH_ELEM_DISP_CALLBACK
dispatch method is configured, you need to pass an event handler by calling touch_xxxx_set_callback()
before the touch element starts working, all the element's extracted message will be passed to the event handler function.
Warning
Since the event handler function runs on the core of the element library, i.e., in the esp_timer callback routine, please avoid performing operations that may cause blocking or delays, such as calling vTaskDelay()
.
In code, the events handle procedure may look like as follows:
/* ---------------------------------------------- TOUCH_ELEM_DISP_EVENT ----------------------------------------------- */
void element_handler_task(void *arg)
{
touch_elem_message_t element_message;
while(1) {
if (touch_element_message_receive(&element_message, Timeout) == ESP_OK) {
const touch_xxxx_message_t *extracted_message = touch_xxxx_get_message(&element_message); //Decode message
... //Event handler logic
}
}
}
void app_main()
{
...
touch_xxxx_set_dispatch_method(element_handle, TOUCH_ELEM_DISP_EVENT); //Set TOUCH_ELEM_DISP_EVENT as the dispatch method
xTaskCreate(&element_handler_task, "element_handler_task", 2048, NULL, 5, NULL); //Create a handler task
...
}
/* -------------------------------------------------------------------------------------------------------------- */
...
/* ---------------------------------------------- TOUCH_ELEM_DISP_CALLBACK ----------------------------------------------- */
void element_handler(touch_xxxx_handle_t out_handle, touch_xxxx_message_t out_message, void *arg)
{
//Event handler logic
}
void app_main()
{
...
touch_xxxx_set_dispatch_method(element_handle, TOUCH_ELEM_DISP_CALLBACK); //Set TOUCH_ELEM_DISP_CALLBACK as the dispatch method
touch_xxxx_set_callback(element_handle, element_handler); //Register an event handler function
...
}
/* -------------------------------------------------------------------------------------------------------------- */
Waterproof Usage
The waterproof shield sensor is always-on after Touch Element waterproof is initialized, however, the waterproof guard sensor is optional, hence if the you do not need the guard sensor,
TOUCH_WATERPROOF_GUARD_NOUSE
has to be passed totouch_element_waterproof_install()
by the configuration struct.To associate the touch element with the guard sensor, pass the touch element's handle to the Touch Element waterproof's masked list by calling
touch_element_waterproof_add()
. By associating a touch element with the Guard sensor, the touch element will be disabled when the guard sensor is triggered by a stream of water so as to protect the touch element.
The Touch Element Waterproof example is available in peripherals/touch_sensor/touch_element/touch_element_waterproof directory.
In code, the waterproof configuration may look as follows:
void app_main()
{
...
touch_xxxx_install(); //Initialize instance (button, slider, etc)
touch_xxxx_create(&element_handle); //Create a new Touch element
...
touch_element_waterproof_install(); //Initialize Touch Element waterproof
touch_element_waterproof_add(element_handle); //Let an element associate with the guard sensor
...
}
Wakeup from Light/Deep-sleep Mode
Only Touch Button can be configured as a wake-up source.
Light- or Deep-sleep modes are both supported to be wakened up by a touch sensor. For the Light-sleep mode, any installed touch button can wake it up. But only the sleep button can wake up from Deep-sleep mode, and the touch sensor will do a calibration immediately, the reference value will be calibrated to a wrong value if our finger does not remove timely. Though the wrong reference value recovers after the finger removes away and has no effect on the driver logic, if you do not want to see a wrong reference value while waking up from Deep-sleep mode, you can call touch_element_sleep_enable_wakeup_calibration()
to disable the wakeup calibration.
The Touch Element Wakeup example is available in system/light_sleep directory.
void app_main()
{
...
touch_element_install();
touch_button_install(); //Initialize the touch button
touch_button_create(&element_handle); //Create a new Touch element
...
// ESP_ERROR_CHECK(touch_element_enable_light_sleep(&sleep_config));
ESP_ERROR_CHECK(touch_element_enable_deep_sleep(button_handle[0], &sleep_config));
// ESP_ERROR_CHECK(touch_element_sleep_enable_wakeup_calibration(button_handle[0], false)); // (optional) Disable wakeup calibration to prevent updating the benchmark to a wrong value
touch_element_start();
...
}
Application Example
All the Touch Element library examples could be found in the peripherals/touch_sensor/touch_element directory of ESP-IDF examples.
API Reference - Touch Element Core
Header File
components/touch_element/include/touch_element/touch_element.h
This header file can be included with:
#include "touch_element/touch_element.h"
This header file is a part of the API provided by the
touch_element
component. To declare that your component depends ontouch_element
, add the following to your CMakeLists.txt:REQUIRES touch_element
or
PRIV_REQUIRES touch_element
Functions
-
esp_err_t touch_element_install(const touch_elem_global_config_t *global_config)
Touch element processing initialization.
Note
To reinitialize the touch element object, call touch_element_uninstall() first
- Parameters
global_config -- [in] Global initialization configuration structure
- Returns
ESP_OK: Successfully initialized
ESP_ERR_INVALID_ARG: Invalid argument
ESP_ERR_NO_MEM: Insufficient memory
ESP_ERR_INVALID_STATE: Touch element is already initialized
Others: Unknown touch driver layer or lower layer error
-
esp_err_t touch_element_start(void)
Touch element processing start.
This function starts the touch element processing system
Note
This function must only be called after all the touch element instances finished creating
- Returns
ESP_OK: Successfully started to process
Others: Unknown touch driver layer or lower layer error
-
esp_err_t touch_element_stop(void)
Touch element processing stop.
This function stops the touch element processing system
Note
This function must be called before changing the system (hardware, software) parameters
- Returns
ESP_OK: Successfully stopped to process
Others: Unknown touch driver layer or lower layer error
-
void touch_element_uninstall(void)
Release resources allocated using touch_element_install.
-
esp_err_t touch_element_message_receive(touch_elem_message_t *element_message, uint32_t ticks_to_wait)
Get current event message of touch element instance.
This function will receive the touch element message (handle, event type, etc...) from te_event_give(). It will block until a touch element event or a timeout occurs.
- Parameters
element_message -- [out] Touch element event message structure
ticks_to_wait -- [in] Number of FreeRTOS ticks to block for waiting event
- Returns
ESP_OK: Successfully received touch element event
ESP_ERR_INVALID_STATE: Touch element library is not initialized
ESP_ERR_INVALID_ARG: element_message is null
ESP_ERR_TIMEOUT: Timed out waiting for event
-
esp_err_t touch_element_waterproof_install(const touch_elem_waterproof_config_t *waterproof_config)
Touch element waterproof initialization.
This function enables the hardware waterproof, then touch element system uses Shield-Sensor and Guard-Sensor to mitigate the influence of water-drop and water-stream.
Note
If the waterproof function is used, Shield-Sensor can not be disabled and it will use channel 14 as it's internal channel. Hence, the user can not use channel 14 for another propose. And the Guard-Sensor is not necessary since it is optional.
Note
Shield-Sensor: It always uses channel 14 as the shield channel, so user must connect the channel 14 and Shield-Layer in PCB since it will generate a synchronous signal automatically
Note
Guard-Sensor: This function is optional. If used, the user must connect the guard channel and Guard-Ring in PCB. Any channels user wants to protect should be added into Guard-Ring in PCB.
- Parameters
waterproof_config -- [in] Waterproof configuration
- Returns
ESP_OK: Successfully initialized
ESP_ERR_INVALID_STATE: Touch element library is not initialized
ESP_ERR_INVALID_ARG: waterproof_config is null or invalid Guard-Sensor channel
ESP_ERR_NO_MEM: Insufficient memory
-
void touch_element_waterproof_uninstall(void)
Release resources allocated using touch_element_waterproof_install()
-
esp_err_t touch_element_waterproof_add(touch_elem_handle_t element_handle)
Add a masked handle to protect while Guard-Sensor has been triggered.
This function will add an application handle (button, slider, etc...) as a masked handle. While Guard-Sensor has been triggered, waterproof function will start working and lock the application internal state. While the influence of water is reduced, the application will be unlock and reset into IDLE state.
Note
The waterproof protection logic must follow the real circuit in PCB, it means that all of the channels inside the input handle must be inside the Guard-Ring in real circuit.
- Parameters
element_handle -- [in] Touch element instance handle
- Returns
ESP_OK: Successfully added a masked handle
ESP_ERR_INVALID_STATE: Waterproof is not initialized
ESP_ERR_INVALID_ARG: element_handle is null
-
esp_err_t touch_element_waterproof_remove(touch_elem_handle_t element_handle)
Remove a masked handle to protect.
This function will remove an application handle from masked handle table.
- Parameters
element_handle -- [in] Touch element instance handle
- Returns
ESP_OK: Successfully removed a masked handle
ESP_ERR_INVALID_STATE: Waterproof is not initialized
ESP_ERR_INVALID_ARG: element_handle is null
ESP_ERR_NOT_FOUND: Failed to search element_handle from waterproof mask_handle list
-
esp_err_t touch_element_enable_light_sleep(const touch_elem_sleep_config_t *sleep_config)
Touch element light sleep initialization.
Note
It should be called after touch button element installed. Any of installed touch element can wake up from the light sleep
- Parameters
sleep_config -- [in] Sleep configurations, set NULL to use default config
- Returns
ESP_OK: Successfully initialized touch sleep
ESP_ERR_INVALID_STATE: Touch element is not installed or touch sleep has been installed
ESP_ERR_INVALID_ARG: inputed argument is NULL
ESP_ERR_NO_MEM: no memory for touch sleep struct
ESP_ERR_NOT_SUPPORTED: inputed wakeup_elem_handle is not touch_button_handle_t type, currently only touch_button_handle_t supported
-
esp_err_t touch_element_disable_light_sleep(void)
Release the resources that allocated by touch_element_enable_deep_sleep()
This function will also disable the touch sensor to wake up the device
- Returns
ESP_OK: uninstall success
ESP_ERR_INVALID_STATE: touch sleep has not been installed
-
esp_err_t touch_element_enable_deep_sleep(touch_elem_handle_t wakeup_elem_handle, const touch_elem_sleep_config_t *sleep_config)
Touch element deep sleep initialization.
This function will enable the device wake-up from deep sleep or light sleep by touch sensor
Note
It should be called after touch button element installed. Only one touch button can be registered as the deep sleep wake-up button
- Parameters
wakeup_elem_handle -- [in] Touch element instance handle for waking up the device, only support button element
sleep_config -- [in] Sleep configurations, set NULL to use default config
- Returns
ESP_OK: Successfully initialized touch sleep
ESP_ERR_INVALID_STATE: Touch element is not installed or touch sleep has been installed
ESP_ERR_INVALID_ARG: inputed argument is NULL
ESP_ERR_NO_MEM: no memory for touch sleep struct
ESP_ERR_NOT_SUPPORTED: inputed wakeup_elem_handle is not touch_button_handle_t type, currently only touch_button_handle_t supported
-
esp_err_t touch_element_disable_deep_sleep(void)
Release the resources that allocated by touch_element_enable_deep_sleep()
This function will also disable the touch sensor to wake up the device
- Returns
ESP_OK: uninstall success
ESP_ERR_INVALID_STATE: touch sleep has not been installed
-
esp_err_t touch_element_sleep_enable_wakeup_calibration(touch_elem_handle_t element_handle, bool en)
Touch element wake up calibrations.
This function will also disable the touch sensor to wake up the device
- Returns
ESP_OK: uninstall success
ESP_ERR_INVALID_STATE: touch sleep has not been installed
Structures
-
struct touch_elem_sw_config_t
Touch element software configuration.
-
struct touch_elem_hw_config_t
Touch element hardware configuration.
Public Members
-
touch_high_volt_t upper_voltage
Touch sensor channel upper charge voltage.
-
touch_volt_atten_t voltage_attenuation
Touch sensor channel upper charge voltage attenuation (Diff voltage is upper - attenuation - lower)
-
touch_low_volt_t lower_voltage
Touch sensor channel lower charge voltage.
-
touch_pad_conn_type_t suspend_channel_polarity
Suspend channel polarity (High Impedance State or GND)
-
touch_pad_denoise_grade_t denoise_level
Internal de-noise level.
-
touch_pad_denoise_cap_t denoise_equivalent_cap
Internal de-noise channel (Touch channel 0) equivalent capacitance.
-
touch_smooth_mode_t smooth_filter_mode
Smooth value filter mode (This only apply to touch_pad_filter_read_smooth())
-
touch_filter_mode_t benchmark_filter_mode
Benchmark filter mode.
-
uint16_t sample_count
The count of sample in each measurement of touch sensor.
-
uint16_t sleep_cycle
The cycle (RTC slow clock) of sleep.
-
uint8_t benchmark_debounce_count
Benchmark debounce count.
-
uint8_t benchmark_calibration_threshold
Benchmark calibration threshold.
-
uint8_t benchmark_jitter_step
Benchmark jitter filter step (This only works at while benchmark filter mode is jitter filter)
-
touch_high_volt_t upper_voltage
-
struct touch_elem_global_config_t
Touch element global configuration passed to touch_element_install.
Public Members
-
touch_elem_hw_config_t hardware
Hardware configuration.
-
touch_elem_sw_config_t software
Software configuration.
-
touch_elem_hw_config_t hardware
-
struct touch_elem_waterproof_config_t
Touch element waterproof configuration passed to touch_element_waterproof_install.
Public Members
-
touch_pad_t guard_channel
Waterproof Guard-Sensor channel number (index)
-
float guard_sensitivity
Waterproof Guard-Sensor sensitivity.
-
touch_pad_t guard_channel
-
struct touch_elem_sleep_config_t
Touch element sleep configuration passed to touch_element_enable_light_sleep or touch_element_enable_deep_sleep.
Public Members
-
uint16_t sample_count
scan times in every measurement, normally equal to the 'sample_count' field in 'touch_elem_hw_config_t'.
-
uint16_t sleep_cycle
sleep_cycle decide the interval between two measurements, t_sleep = sleep_cycle / (RTC_SLOW_CLK frequency), normally equal to the 'sleep_cycle' field in 'touch_elem_hw_config_t'.
-
uint16_t sample_count
-
struct touch_elem_message_t
Touch element event message type from touch_element_message_receive()
Public Members
-
touch_elem_handle_t handle
Touch element handle.
-
touch_elem_type_t element_type
Touch element type.
-
void *arg
User input argument.
-
uint8_t child_msg[8]
Encoded message.
-
touch_elem_handle_t handle
Macros
-
TOUCH_ELEM_GLOBAL_DEFAULT_CONFIG()
-
TOUCH_ELEM_EVENT_NONE
None event.
-
TOUCH_ELEM_EVENT_ON_PRESS
On Press event.
-
TOUCH_ELEM_EVENT_ON_RELEASE
On Release event.
-
TOUCH_ELEM_EVENT_ON_LONGPRESS
On LongPress event.
-
TOUCH_ELEM_EVENT_ON_CALCULATION
On Calculation event.
-
TOUCH_WATERPROOF_GUARD_NOUSE
Waterproof no use guard sensor.
Type Definitions
-
typedef void *touch_elem_handle_t
Touch element handle type.
-
typedef uint32_t touch_elem_event_t
Touch element event type.
Enumerations
API Reference - Touch Slider
Header File
components/touch_element/include/touch_element/touch_slider.h
This header file can be included with:
#include "touch_element/touch_slider.h"
This header file is a part of the API provided by the
touch_element
component. To declare that your component depends ontouch_element
, add the following to your CMakeLists.txt:REQUIRES touch_element
or
PRIV_REQUIRES touch_element
Functions
-
esp_err_t touch_slider_install(const touch_slider_global_config_t *global_config)
Touch slider initialize.
This function initializes touch slider object and acts on all touch slider instances.
- Parameters
global_config -- [in] Touch slider global initialization configuration
- Returns
ESP_OK: Successfully initialized touch slider
ESP_ERR_INVALID_STATE: Touch element library was not initialized
ESP_ERR_INVALID_ARG: slider_init is NULL
ESP_ERR_NO_MEM: Insufficient memory
-
void touch_slider_uninstall(void)
Release resources allocated using touch_slider_install()
-
esp_err_t touch_slider_create(const touch_slider_config_t *slider_config, touch_slider_handle_t *slider_handle)
Create a new touch slider instance.
Note
The index of Channel array and sensitivity array must be one-one correspondence
- Parameters
slider_config -- [in] Slider configuration
slider_handle -- [out] Slider handle
- Returns
ESP_OK: Successfully create touch slider
ESP_ERR_INVALID_STATE: Touch slider driver was not initialized
ESP_ERR_INVALID_ARG: Invalid configuration struct or arguments is NULL
ESP_ERR_NO_MEM: Insufficient memory
-
esp_err_t touch_slider_delete(touch_slider_handle_t slider_handle)
Release resources allocated using touch_slider_create.
- Parameters
slider_handle -- [in] Slider handle
- Returns
ESP_OK: Successfully released resources
ESP_ERR_INVALID_STATE: Touch slider driver was not initialized
ESP_ERR_INVALID_ARG: slider_handle is null
ESP_ERR_NOT_FOUND: Input handle is not a slider handle
-
esp_err_t touch_slider_subscribe_event(touch_slider_handle_t slider_handle, uint32_t event_mask, void *arg)
Touch slider subscribes event.
This function uses event mask to subscribe to touch slider events, once one of the subscribed events occurs, the event message could be retrieved by calling touch_element_message_receive() or input callback routine.
Note
Touch slider only support three kind of event masks, they are TOUCH_ELEM_EVENT_ON_PRESS, TOUCH_ELEM_EVENT_ON_RELEASE. You can use those event masks in any combination to achieve the desired effect.
- Parameters
slider_handle -- [in] Slider handle
event_mask -- [in] Slider subscription event mask
arg -- [in] User input argument
- Returns
ESP_OK: Successfully subscribed touch slider event
ESP_ERR_INVALID_STATE: Touch slider driver was not initialized
ESP_ERR_INVALID_ARG: slider_handle is null or event is not supported
-
esp_err_t touch_slider_set_dispatch_method(touch_slider_handle_t slider_handle, touch_elem_dispatch_t dispatch_method)
Touch slider set dispatch method.
This function sets a dispatch method that the driver core will use this method as the event notification method.
- Parameters
slider_handle -- [in] Slider handle
dispatch_method -- [in] Dispatch method (By callback/event)
- Returns
ESP_OK: Successfully set dispatch method
ESP_ERR_INVALID_STATE: Touch slider driver was not initialized
ESP_ERR_INVALID_ARG: slider_handle is null or dispatch_method is invalid
-
esp_err_t touch_slider_set_callback(touch_slider_handle_t slider_handle, touch_slider_callback_t slider_callback)
Touch slider set callback.
This function sets a callback routine into touch element driver core, when the subscribed events occur, the callback routine will be called.
Note
Slider message will be passed from the callback function and it will be destroyed when the callback function return.
Warning
Since this input callback routine runs on driver core (esp-timer callback routine), it should not do something that attempts to Block, such as calling vTaskDelay().
- Parameters
slider_handle -- [in] Slider handle
slider_callback -- [in] User input callback
- Returns
ESP_OK: Successfully set callback
ESP_ERR_INVALID_STATE: Touch slider driver was not initialized
ESP_ERR_INVALID_ARG: slider_handle or slider_callback is null
-
const touch_slider_message_t *touch_slider_get_message(const touch_elem_message_t *element_message)
Touch slider get message.
This function decodes the element message from touch_element_message_receive() and return a slider message pointer.
- Parameters
element_message -- [in] element message
- Returns
Touch slider message pointer
Structures
-
struct touch_slider_global_config_t
Slider initialization configuration passed to touch_slider_install.
Public Members
-
float quantify_lower_threshold
Slider signal quantification threshold.
-
float threshold_divider
Slider channel threshold divider.
-
uint16_t filter_reset_time
Slider position filter reset time (Unit is esp_timer callback tick)
-
uint16_t benchmark_update_time
Slider benchmark update time (Unit is esp_timer callback tick)
-
uint8_t position_filter_size
Moving window filter buffer size.
-
uint8_t position_filter_factor
One-order IIR filter factor.
-
uint8_t calculate_channel_count
The number of channels which will take part in calculation.
-
float quantify_lower_threshold
-
struct touch_slider_config_t
Slider configuration (for new instance) passed to touch_slider_create()
Public Members
-
const touch_pad_t *channel_array
Slider channel array.
-
const float *sensitivity_array
Slider channel sensitivity array.
-
uint8_t channel_num
The number of slider channels.
-
uint8_t position_range
The right region of touch slider position range, [0, position_range (less than or equal to 255)].
-
const touch_pad_t *channel_array
-
struct touch_slider_message_t
Slider message type.
Public Members
-
touch_slider_event_t event
Slider event.
-
touch_slider_position_t position
Slider position.
-
touch_slider_event_t event
Macros
-
TOUCH_SLIDER_GLOBAL_DEFAULT_CONFIG()
Type Definitions
-
typedef uint32_t touch_slider_position_t
Slider position data type.
-
typedef touch_elem_handle_t touch_slider_handle_t
Slider instance handle.
-
typedef void (*touch_slider_callback_t)(touch_slider_handle_t, touch_slider_message_t*, void*)
Slider callback type.
Enumerations
API Reference - Touch Matrix
Header File
components/touch_element/include/touch_element/touch_matrix.h
This header file can be included with:
#include "touch_element/touch_matrix.h"
This header file is a part of the API provided by the
touch_element
component. To declare that your component depends ontouch_element
, add the following to your CMakeLists.txt:REQUIRES touch_element
or
PRIV_REQUIRES touch_element
Functions
-
esp_err_t touch_matrix_install(const touch_matrix_global_config_t *global_config)
Touch matrix button initialize.
This function initializes touch matrix button object and acts on all touch matrix button instances.
- Parameters
global_config -- [in] Touch matrix global initialization configuration
- Returns
ESP_OK: Successfully initialized touch matrix button
ESP_ERR_INVALID_STATE: Touch element library was not initialized
ESP_ERR_INVALID_ARG: matrix_init is NULL
ESP_ERR_NO_MEM: Insufficient memory
-
void touch_matrix_uninstall(void)
Release resources allocated using touch_matrix_install()
-
esp_err_t touch_matrix_create(const touch_matrix_config_t *matrix_config, touch_matrix_handle_t *matrix_handle)
Create a new touch matrix button instance.
Note
Channel array and sensitivity array must be one-one correspondence in those array
Note
Touch matrix button does not support Multi-Touch now
- Parameters
matrix_config -- [in] Matrix button configuration
matrix_handle -- [out] Matrix button handle
- Returns
ESP_OK: Successfully create touch matrix button
ESP_ERR_INVALID_STATE: Touch matrix driver was not initialized
ESP_ERR_INVALID_ARG: Invalid configuration struct or arguments is NULL
ESP_ERR_NO_MEM: Insufficient memory
-
esp_err_t touch_matrix_delete(touch_matrix_handle_t matrix_handle)
Release resources allocated using touch_matrix_create()
- Parameters
matrix_handle -- [in] Matrix handle
- Returns
ESP_OK: Successfully released resources
ESP_ERR_INVALID_STATE: Touch matrix driver was not initialized
ESP_ERR_INVALID_ARG: matrix_handle is null
ESP_ERR_NOT_FOUND: Input handle is not a matrix handle
-
esp_err_t touch_matrix_subscribe_event(touch_matrix_handle_t matrix_handle, uint32_t event_mask, void *arg)
Touch matrix button subscribes event.
This function uses event mask to subscribe to touch matrix events, once one of the subscribed events occurs, the event message could be retrieved by calling touch_element_message_receive() or input callback routine.
Note
Touch matrix button only support three kind of event masks, they are TOUCH_ELEM_EVENT_ON_PRESS, TOUCH_ELEM_EVENT_ON_RELEASE, TOUCH_ELEM_EVENT_ON_LONGPRESS. You can use those event masks in any combination to achieve the desired effect.
- Parameters
matrix_handle -- [in] Matrix handle
event_mask -- [in] Matrix subscription event mask
arg -- [in] User input argument
- Returns
ESP_OK: Successfully subscribed touch matrix event
ESP_ERR_INVALID_STATE: Touch matrix driver was not initialized
ESP_ERR_INVALID_ARG: matrix_handle is null or event is not supported
-
esp_err_t touch_matrix_set_dispatch_method(touch_matrix_handle_t matrix_handle, touch_elem_dispatch_t dispatch_method)
Touch matrix button set dispatch method.
This function sets a dispatch method that the driver core will use this method as the event notification method.
- Parameters
matrix_handle -- [in] Matrix button handle
dispatch_method -- [in] Dispatch method (By callback/event)
- Returns
ESP_OK: Successfully set dispatch method
ESP_ERR_INVALID_STATE: Touch matrix driver was not initialized
ESP_ERR_INVALID_ARG: matrix_handle is null or dispatch_method is invalid
-
esp_err_t touch_matrix_set_callback(touch_matrix_handle_t matrix_handle, touch_matrix_callback_t matrix_callback)
Touch matrix button set callback.
This function sets a callback routine into touch element driver core, when the subscribed events occur, the callback routine will be called.
Note
Matrix message will be passed from the callback function and it will be destroyed when the callback function return.
Warning
Since this input callback routine runs on driver core (esp-timer callback routine), it should not do something that attempts to Block, such as calling vTaskDelay().
- Parameters
matrix_handle -- [in] Matrix button handle
matrix_callback -- [in] User input callback
- Returns
ESP_OK: Successfully set callback
ESP_ERR_INVALID_STATE: Touch matrix driver was not initialized
ESP_ERR_INVALID_ARG: matrix_handle or matrix_callback is null
-
esp_err_t touch_matrix_set_longpress(touch_matrix_handle_t matrix_handle, uint32_t threshold_time)
Touch matrix button set long press trigger time.
This function sets the threshold time (ms) for a long press event. If a matrix button is pressed and held for a period of time that exceeds the threshold time, a long press event is triggered.
- Parameters
matrix_handle -- [in] Matrix button handle
threshold_time -- [in] Threshold time (ms) of long press event occur
- Returns
ESP_OK: Successfully set the time of long press event
ESP_ERR_INVALID_STATE: Touch matrix driver was not initialized
ESP_ERR_INVALID_ARG: matrix_handle is null or time (ms) is 0
-
const touch_matrix_message_t *touch_matrix_get_message(const touch_elem_message_t *element_message)
Touch matrix get message.
This function decodes the element message from touch_element_message_receive() and return a matrix message pointer.
- Parameters
element_message -- [in] element message
- Returns
Touch matrix message pointer
Structures
-
struct touch_matrix_global_config_t
Matrix button initialization configuration passed to touch_matrix_install.
-
struct touch_matrix_config_t
Matrix button configuration (for new instance) passed to touch_matrix_create()
Public Members
-
const touch_pad_t *x_channel_array
Matrix button x-axis channels array.
-
const touch_pad_t *y_channel_array
Matrix button y-axis channels array.
-
const float *x_sensitivity_array
Matrix button x-axis channels sensitivity array.
-
const float *y_sensitivity_array
Matrix button y-axis channels sensitivity array.
-
uint8_t x_channel_num
The number of channels in x-axis.
-
uint8_t y_channel_num
The number of channels in y-axis.
-
const touch_pad_t *x_channel_array
-
struct touch_matrix_position_t
Matrix button position data type.
-
struct touch_matrix_message_t
Matrix message type.
Public Members
-
touch_matrix_event_t event
Matrix event.
-
touch_matrix_position_t position
Matrix position.
-
touch_matrix_event_t event
Macros
-
TOUCH_MATRIX_GLOBAL_DEFAULT_CONFIG()
Type Definitions
-
typedef touch_elem_handle_t touch_matrix_handle_t
Matrix button instance handle.
-
typedef void (*touch_matrix_callback_t)(touch_matrix_handle_t, touch_matrix_message_t*, void*)
Matrix button callback type.