集成电路总线 (I2C)
简介
I2C 是一种串行同步半双工通信协议,总线上可以同时挂载多个主机和从机。I2C 使用两条双向开漏线:串行数据线 (SDA) 和串行时钟线 (SCL),通过电阻上拉。
ESP32-C3 有 1 个 I2C 控制器(也被称为端口),负责处理 I2C 总线上的通信。
单个 I2C 控制器既可以是主机也可以是从机。
通常,I2C 从机设备具有 7 位地址或 10 位地址。ESP32-C3 支持 I2C 标准模式 (Sm) 和快速模式 (Fm),可分别达到 100 kHz 和 400 kHz。
警告
主机模式的 SCL 时钟频率不应大于 400 kHz。
备注
SCL 的频率受上拉电阻及导线电容的影响。因此,强烈建议选择适当的上拉电阻,确保频率准确。推荐的上拉电阻值通常在 1 kΩ 到 10 kΩ 之间。
请注意,SCL 的频率越高,上拉电阻应该越小(但不能小于 1 kΩ)。较大的电阻会降低电流,增加时钟切换时间并降低频率。通常推荐 2 kΩ 到 5 kΩ 左右的电阻,也可根据电流需求进行一定调整。
I2C 时钟配置
i2c_clock_source_t::I2C_CLK_SRC_DEFAULT
:默认的 I2C 时钟源。i2c_clock_source_t::I2C_CLK_SRC_XTAL
:以外部晶振作为 I2C 时钟源。i2c_clock_source_t::I2C_CLK_RC_FAST
:以内部 20 MHz RC 振荡器作为 I2C 时钟源。
I2C 文件结构
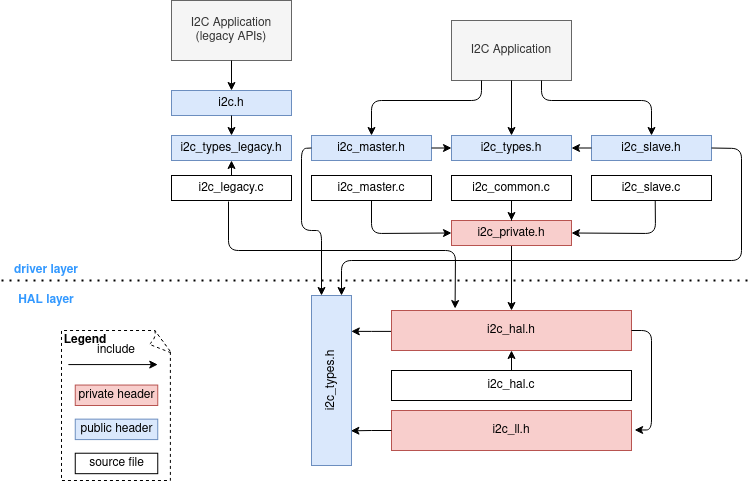
I2C 文件结构
需要包含在 I2C 应用程序中的公共头文件
i2c.h
:遗留 I2C API 的头文件(用于使用旧驱动程序的应用)。i2c_master.h
:提供标准通信模式下特定 API 的头文件(用于使用主机模式的新驱动程序的应用)。i2c_slave.h
:提供标准通信模式下特定 API 的头文件(用于使从机模式的新驱动程序的应用)。
备注
旧驱动程序与新驱动程序无法共存。包含 i2c.h
头文件可使用旧驱动程序,或包含 i2c_master.h
和 i2c_slave.h
来使用新驱动程序。请注意,现已弃用旧驱动程序,之后将移除。
上述头文件中包含的公共头文件
i2c_types_legacy.h
:仅在旧驱动程序中使用的旧公共类型。i2c_types.h
:提供公共类型的头文件。
功能概述
I2C 驱动程序提供以下服务:
资源分配 - 包括如何使用正确的配置来分配 I2C 总线,以及如何在完成工作后回收资源。
I2C 主机控制器 - 包括 I2C 主机控制器的行为,介绍了数据发送、数据接收和数据的双向传输。
I2C 从机控制器 - 包括 I2C 从机控制器的行为,涉及数据发送和数据接收。
电源管理 - 描述了不同时钟源对功耗的影响。
IRAM 安全 - 描述了如何在 cache 被禁用时正常运行 I2C 中断。
线程安全 - 列出了驱动程序中线程安全的 API。
Kconfig 选项 - 列出了支持的 Kconfig 选项,这些选项可以对驱动程序产生不同影响。
资源分配
若系统支持 I2C 主机总线和 I2C 从机总线,则由驱动程序中的 i2c_bus_handle_t
来表示。资源池管理可用的端口,并在有请求时分配空闲端口。
安装 I2C 主机总线和设备
I2C 主机总线是基于总线-设备模型设计的,因此需要分别使用 i2c_master_bus_config_t
和 i2c_device_config_t
来分配 I2C 主机总线实例和 I2C 设备实例。
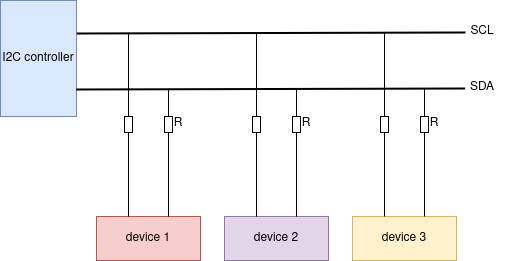
I2C 主机总线-设备模块
I2C 主机总线需要 i2c_master_bus_config_t
指定的配置:
i2c_master_bus_config_t::i2c_port
设置控制器使用的 I2C 端口。i2c_master_bus_config_t::sda_io_num
设置串行数据总线 (SDA) 的 GPIO 编号。i2c_master_bus_config_t::scl_io_num
设置串行时钟总线 (SCL) 的 GPIO 编号。i2c_master_bus_config_t::clk_source
选择 I2C 总线的时钟源。可用时钟列表见i2c_clock_source_t
。有关不同时钟源对功耗的影响,请参阅 电源管理 部分。i2c_master_bus_config_t::glitch_ignore_cnt
设置主机总线的毛刺周期。如果线上的毛刺周期小于设置的值(通常设为 7),则可以被滤除。i2c_master_bus_config_t::intr_priority
设置中断的优先级。如果设置为0
,则驱动程序将使用低或中优先级的中断(优先级可设为 1、2 或 3 中的一个),若未设置,则将使用i2c_master_bus_config_t::intr_priority
指示的优先级。请使用数字形式(1、2、3),不要用位掩码形式((1<<1)、(1<<2)、(1<<3))。i2c_master_bus_config_t::trans_queue_depth
设置内部传输队列的深度,但仅在异步传输中有效。i2c_master_bus_config_t::enable_internal_pullup
启用内部上拉电阻。注意:该设置无法在高速频率下拉高总线,此时建议使用合适的外部上拉电阻。
如果在 i2c_master_bus_config_t
中指定了配置,则可调用 i2c_new_master_bus()
来分配和初始化 I2C 主机总线。如果函数运行正确,则将返回一个 I2C 总线句柄。若没有可用的 I2C 端口,此函数将返回 ESP_ERR_NOT_FOUND
错误。
I2C 主机设备需要 i2c_device_config_t
指定的配置:
i2c_device_config_t::dev_addr_length
配置从机设备的地址位长度,可从枚举I2C_ADDR_BIT_LEN_7
或I2C_ADDR_BIT_LEN_10
(如果支持)中进行选择。i2c_device_config_t::device_address
设置 I2C 设备原始地址,请直接将设备地址解析到此成员。例如,若设备地址为 0x28,则将 0x28 解析到i2c_device_config_t::device_address
,不要带写入或读取位。i2c_device_config_t::scl_speed_hz
设置此设备的 SCL 线频率。i2c_device_config_t::scl_wait_us
设置 SCL 等待时间(以微秒为单位)。通常此值较大,因为从机延伸时间会很长(甚至可能延伸到 12 ms)。设置为0
表示使用默认的寄存器值。
一旦填充好 i2c_device_config_t
结构体的必要参数,就可调用 i2c_master_bus_add_device()
来分配 I2C 设备实例,并将设备挂载到主机总线上。如果函数运行正确,则将返回一个 I2C 设备句柄。若未正确初始化 I2C 总线,此函数将返回 ESP_ERR_INVALID_ARG
错误。
#include "driver/i2c_master.h"
i2c_master_bus_config_t i2c_mst_config = {
.clk_source = I2C_CLK_SRC_DEFAULT,
.i2c_port = TEST_I2C_PORT,
.scl_io_num = I2C_MASTER_SCL_IO,
.sda_io_num = I2C_MASTER_SDA_IO,
.glitch_ignore_cnt = 7,
.flags.enable_internal_pullup = true,
};
i2c_master_bus_handle_t bus_handle;
ESP_ERROR_CHECK(i2c_new_master_bus(&i2c_mst_config, &bus_handle));
i2c_device_config_t dev_cfg = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x58,
.scl_speed_hz = 100000,
};
i2c_master_dev_handle_t dev_handle;
ESP_ERROR_CHECK(i2c_master_bus_add_device(bus_handle, &dev_cfg, &dev_handle));
卸载 I2C 主机总线和设备
如果不再需要之前安装的 I2C 总线或设备,建议调用 i2c_master_bus_rm_device()
或 i2c_del_master_bus()
来回收资源,以释放底层硬件。
安装 I2C 从机设备
I2C 从机设备需要 i2c_slave_config_t
指定的配置:
i2c_slave_config_t::i2c_port
设置控制器使用的 I2C 端口。i2c_slave_config_t::sda_io_num
设置串行数据总线 (SDA) 的 GPIO 编号。i2c_slave_config_t::scl_io_num
设置串行时钟总线 (SCL) 的 GPIO 编号。i2c_slave_config_t::clk_source
选择 I2C 总线的时钟源。可用时钟列表见i2c_clock_source_t
。有关不同时钟源对功耗的影响,请参阅 电源管理。i2c_slave_config_t::send_buf_depth
设置发送 buffer 的长度。i2c_slave_config_t::slave_addr
设置从机地址。i2c_master_bus_config_t::intr_priority
设置中断的优先级。如果设置为0
,则驱动程序将使用低或中优先级的中断(优先级可设为 1、2 或 3 中的一个),若未设置,则将使用i2c_master_bus_config_t::intr_priority
指示的优先级。请使用数字形式(1、2、3),不要用位掩码形式((1<<1)、(1<<2)、(1<<3))。请注意,中断优先级一旦设置完成,在调用i2c_del_master_bus()
之前都无法更改。i2c_slave_config_t::addr_bit_len
。如果需要从机设备具有 10 位地址,则将该成员变量设为I2C_ADDR_BIT_LEN_10
。i2c_slave_config_t::stretch_en
。如果要启用从机控制器拉伸功能,请将该成员变量设为 true。有关 I2C 拉伸的工作原理,请参阅 [TRM]。i2c_slave_config_t::broadcast_en
。如果要启用从机广播,请将该成员变量设为 true。当从机设备接收到来自主机设备的通用调用地址 0x00,且后面的读写位为 0 时,无论从机设备自身地址如何,都会响应主机设备。i2c_slave_config_t::access_ram_en
。如果要启用 non-FIFO 模式,请将该成员变量设为 true,则 I2C 数据 FIFO 可用作 RAM,并将同步打开双地址。
一旦填充好 i2c_slave_config_t
结构体的必要参数,就可调用 i2c_new_slave_device()
来分配和初始化 I2C 主机总线。如果函数运行正确,则将返回一个 I2C 总线句柄。若没有可用的 I2C 端口,此函数将返回 ESP_ERR_NOT_FOUND
错误。
i2c_slave_config_t i2c_slv_config = {
.addr_bit_len = I2C_ADDR_BIT_LEN_7,
.clk_source = I2C_CLK_SRC_DEFAULT,
.i2c_port = TEST_I2C_PORT,
.send_buf_depth = 256,
.scl_io_num = I2C_SLAVE_SCL_IO,
.sda_io_num = I2C_SLAVE_SDA_IO,
.slave_addr = 0x58,
};
i2c_slave_dev_handle_t slave_handle;
ESP_ERROR_CHECK(i2c_new_slave_device(&i2c_slv_config, &slave_handle));
卸载 I2C 从机设备
如果不再需要之前安装的 I2C 总线,建议调用 i2c_del_slave_device()
来回收资源,以释放底层硬件。
I2C 主机控制器
通过调用 i2c_new_master_bus()
安装好 I2C 主机控制器驱动程序后,ESP32-C3 就可以与其他 I2C 设备进行通信了。I2C API 允许标准事务,如下图所示:
I2C 主机写入
在成功安装 I2C 主机总线之后,可以通过调用 i2c_master_transmit()
来向从机设备写入数据。下图解释了该函数的原理。
简单来说,驱动程序用一系列命令填充了一个命令链,并将该命令链传递给 I2C 控制器执行。

I2C 主机向从机设备写入数据
将数据写入从机设备的简单示例:
#define DATA_LENGTH 100
i2c_master_bus_config_t i2c_mst_config = {
.clk_source = I2C_CLK_SRC_DEFAULT,
.i2c_port = I2C_PORT_NUM_0,
.scl_io_num = I2C_MASTER_SCL_IO,
.sda_io_num = I2C_MASTER_SDA_IO,
.glitch_ignore_cnt = 7,
};
i2c_master_bus_handle_t bus_handle;
ESP_ERROR_CHECK(i2c_new_master_bus(&i2c_mst_config, &bus_handle));
i2c_device_config_t dev_cfg = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x58,
.scl_speed_hz = 100000,
};
i2c_master_dev_handle_t dev_handle;
ESP_ERROR_CHECK(i2c_master_bus_add_device(bus_handle, &dev_cfg, &dev_handle));
ESP_ERROR_CHECK(i2c_master_transmit(dev_handle, data_wr, DATA_LENGTH, -1));
I2C 主机写入操作还支持在单次传输事务中传输多个 buffer。如下所示:
uint8_t control_phase_byte = 0;
size_t control_phase_size = 0;
if (/*condition*/) {
control_phase_byte = 1;
control_phase_size = 1;
}
uint8_t *cmd_buffer = NULL;
size_t cmd_buffer_size = 0;
if (/*condition*/) {
uint8_t cmds[4] = {BYTESHIFT(lcd_cmd, 3), BYTESHIFT(lcd_cmd, 2), BYTESHIFT(lcd_cmd, 1), BYTESHIFT(lcd_cmd, 0)};
cmd_buffer = cmds;
cmd_buffer_size = 4;
}
uint8_t *lcd_buffer = NULL;
size_t lcd_buffer_size = 0;
if (buffer) {
lcd_buffer = (uint8_t*)buffer;
lcd_buffer_size = buffer_size;
}
i2c_master_transmit_multi_buffer_info_t lcd_i2c_buffer[3] = {
{.write_buffer = &control_phase_byte, .buffer_size = control_phase_size},
{.write_buffer = cmd_buffer, .buffer_size = cmd_buffer_size},
{.write_buffer = lcd_buffer, .buffer_size = lcd_buffer_size},
};
i2c_master_multi_buffer_transmit(handle, lcd_i2c_buffer, sizeof(lcd_i2c_buffer) / sizeof(i2c_master_transmit_multi_buffer_info_t), -1);
I2C 主机读取
在成功安装 I2C 主机总线后,可以通过调用 i2c_master_receive()
从从机设备读取数据。下图解释了该函数的原理。

I2C 主机从从机设备读取数据
从从机设备读取数据的简单示例:
#define DATA_LENGTH 100
i2c_master_bus_config_t i2c_mst_config = {
.clk_source = I2C_CLK_SRC_DEFAULT,
.i2c_port = I2C_PORT_NUM_0,
.scl_io_num = I2C_MASTER_SCL_IO,
.sda_io_num = I2C_MASTER_SDA_IO,
.glitch_ignore_cnt = 7,
};
i2c_master_bus_handle_t bus_handle;
ESP_ERROR_CHECK(i2c_new_master_bus(&i2c_mst_config, &bus_handle));
i2c_device_config_t dev_cfg = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x58,
.scl_speed_hz = 100000,
};
i2c_master_dev_handle_t dev_handle;
ESP_ERROR_CHECK(i2c_master_bus_add_device(bus_handle, &dev_cfg, &dev_handle));
i2c_master_receive(dev_handle, data_rd, DATA_LENGTH, -1);
I2C 主机写入后读取
从一些 I2C 设备中读取数据之前需要进行写入配置,可通过 i2c_master_transmit_receive()
接口进行配置。下图解释了该函数的原理。

I2C 主机向从机设备写入并从从机设备读取数据
请注意,在写入操作和读取操作之间没有插入 STOP 条件位,因此该功能适用于从 I2C 设备读取寄存器。以下是向从机设备写入数据并从从机设备读取数据的简单示例:
i2c_device_config_t dev_cfg = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x58,
.scl_speed_hz = 100000,
};
i2c_master_dev_handle_t dev_handle;
ESP_ERROR_CHECK(i2c_master_bus_add_device(I2C_PORT_NUM_0, &dev_cfg, &dev_handle));
uint8_t buf[20] = {0x20};
uint8_t buffer[2];
ESP_ERROR_CHECK(i2c_master_transmit_receive(dev_handle, buf, sizeof(buf), buffer, 2, -1));
I2C 主机探测
I2C 驱动程序可以使用 i2c_master_probe()
来检测设备是否已经连接到 I2C 总线上。如果该函数返回 ESP_OK
,则表示该设备已经被检测到。
重要
在调用该函数时,必须将上拉电阻连接到 SCL 和 SDA 管脚。如果在正确解析 xfer_timeout_ms 时收到 ESP_ERR_TIMEOUT,则应检查上拉电阻。若暂无合适的电阻,也可将 flags.enable_internal_pullup 设为 true。

I2C 主机探测
探测 I2C 设备的简单示例:
i2c_master_bus_config_t i2c_mst_config_1 = {
.clk_source = I2C_CLK_SRC_DEFAULT,
.i2c_port = TEST_I2C_PORT,
.scl_io_num = I2C_MASTER_SCL_IO,
.sda_io_num = I2C_MASTER_SDA_IO,
.glitch_ignore_cnt = 7,
.flags.enable_internal_pullup = true,
};
i2c_master_bus_handle_t bus_handle;
ESP_ERROR_CHECK(i2c_new_master_bus(&i2c_mst_config_1, &bus_handle));
ESP_ERROR_CHECK(i2c_master_probe(bus_handle, 0x22, -1));
ESP_ERROR_CHECK(i2c_del_master_bus(bus_handle));
I2C 从机控制器
通过调用 i2c_new_slave_device()
安装好 I2C 从机驱动程序后,ESP32-C3 就可以作为从机与其他 I2C 主机进行通信了。
I2C 从机写入
I2C 从机的发送 buffer 可作为 FIFO 来存储要发送的数据。在主机请求这些数据前,它们会一直排队。可通过调用 i2c_slave_transmit()
来传输数据。
将数据写入 FIFO 的简单示例:
uint8_t *data_wr = (uint8_t *) malloc(DATA_LENGTH);
i2c_slave_config_t i2c_slv_config = {
.addr_bit_len = I2C_ADDR_BIT_LEN_7, // 7 位地址
.clk_source = I2C_CLK_SRC_DEFAULT, // 设置时钟源
.i2c_port = 0, // 设置 I2C 端口编号
.send_buf_depth = 256, // 设置 TX buffer 长度
.scl_io_num = 2, // SCL 管脚编号
.sda_io_num = 1, // SDA 管脚编号
.slave_addr = 0x58, // 从机地址
};
i2c_bus_handle_t i2c_bus_handle;
ESP_ERROR_CHECK(i2c_new_slave_device(&i2c_slv_config, &i2c_bus_handle));
for (int i = 0; i < DATA_LENGTH; i++) {
data_wr[i] = i;
}
ESP_ERROR_CHECK(i2c_slave_transmit(i2c_bus_handle, data_wr, DATA_LENGTH, 10000));
I2C 从机读取
每当主机将数据写入从机,从机都会自动将数据存储在接收 buffer 中,从而使从机应用程序能自由调用 i2c_slave_receive()
。i2c_slave_receive()
为非阻塞接口,因此要想知道接收是否完成,需注册回调函数 i2c_slave_register_event_callbacks()
。
static IRAM_ATTR bool i2c_slave_rx_done_callback(i2c_slave_dev_handle_t channel, const i2c_slave_rx_done_event_data_t *edata, void *user_data)
{
BaseType_t high_task_wakeup = pdFALSE;
QueueHandle_t receive_queue = (QueueHandle_t)user_data;
xQueueSendFromISR(receive_queue, edata, &high_task_wakeup);
return high_task_wakeup == pdTRUE;
}
uint8_t *data_rd = (uint8_t *) malloc(DATA_LENGTH);
uint32_t size_rd = 0;
i2c_slave_config_t i2c_slv_config = {
.addr_bit_len = I2C_ADDR_BIT_LEN_7,
.clk_source = I2C_CLK_SRC_DEFAULT,
.i2c_port = TEST_I2C_PORT,
.send_buf_depth = 256,
.scl_io_num = I2C_SLAVE_SCL_IO,
.sda_io_num = I2C_SLAVE_SDA_IO,
.slave_addr = 0x58,
};
i2c_slave_dev_handle_t slave_handle;
ESP_ERROR_CHECK(i2c_new_slave_device(&i2c_slv_config, &slave_handle));
s_receive_queue = xQueueCreate(1, sizeof(i2c_slave_rx_done_event_data_t));
i2c_slave_event_callbacks_t cbs = {
.on_recv_done = i2c_slave_rx_done_callback,
};
ESP_ERROR_CHECK(i2c_slave_register_event_callbacks(slave_handle, &cbs, s_receive_queue));
i2c_slave_rx_done_event_data_t rx_data;
ESP_ERROR_CHECK(i2c_slave_receive(slave_handle, data_rd, DATA_LENGTH));
xQueueReceive(s_receive_queue, &rx_data, pdMS_TO_TICKS(10000));
// 接收完成。
将数据放入 I2C 从机 RAM 中
如上所述,I2C 从机 FIFO 可被用作 RAM,即可以通过地址字段直接访问 RAM。例如,可参照下图将数据写入第三个 RAM 块。请注意,在进行操作前需要先将 i2c_slave_config_t::access_ram_en
设为 true。

将数据放入 I2C 从机 RAM 中
uint8_t data_rd[DATA_LENGTH_RAM] = {0};
i2c_slave_config_t i2c_slv_config = {
.addr_bit_len = I2C_ADDR_BIT_LEN_7,
.clk_source = I2C_CLK_SRC_DEFAULT,
.i2c_port = TEST_I2C_PORT,
.send_buf_depth = 256,
.scl_io_num = I2C_SLAVE_SCL_IO,
.sda_io_num = I2C_SLAVE_SDA_IO,
.slave_addr = 0x58,
.flags.access_ram_en = true,
};
// 主机将数据写入从机。
i2c_slave_dev_handle_t slave_handle;
ESP_ERROR_CHECK(i2c_new_slave_device(&i2c_slv_config, &slave_handle));
ESP_ERROR_CHECK(i2c_slave_read_ram(slave_handle, 0x5, data_rd, DATA_LENGTH_RAM));
ESP_ERROR_CHECK(i2c_del_slave_device(slave_handle));
从 I2C 从机 RAM 中获取数据
数据可被存储在相对从机一定偏移量的 RAM 中,且主机可直接通过 RAM 地址读取这些数据。例如,如果数据被存储在第三个 RAM 块中,则主机可参照下图读取这些数据。请注意,在操作前需要先将 i2c_slave_config_t::access_ram_en
设为 true。

从 I2C 从机 RAM 中获取数据
uint8_t data_wr[DATA_LENGTH_RAM] = {0};
i2c_slave_config_t i2c_slv_config = {
.addr_bit_len = I2C_ADDR_BIT_LEN_7,
.clk_source = I2C_CLK_SRC_DEFAULT,
.i2c_port = TEST_I2C_PORT,
.send_buf_depth = 256,
.scl_io_num = I2C_SLAVE_SCL_IO,
.sda_io_num = I2C_SLAVE_SDA_IO,
.slave_addr = 0x58,
.flags.access_ram_en = true,
};
i2c_slave_dev_handle_t slave_handle;
ESP_ERROR_CHECK(i2c_new_slave_device(&i2c_slv_config, &slave_handle));
ESP_ERROR_CHECK(i2c_slave_write_ram(slave_handle, 0x2, data_wr, DATA_LENGTH_RAM));
ESP_ERROR_CHECK(i2c_del_slave_device(slave_handle));
注册事件回调函数
I2C 主机回调
当 I2C 主机总线触发中断时,将生成特定事件并通知 CPU。如果需要在发生这些事件时调用某些函数,可通过 i2c_master_register_event_callbacks()
将这些函数挂接到中断服务程序 (ISR) 上。由于注册的回调函数是在中断上下文中被调用的,所以应确保这些函数不会阻塞(例如,确保仅从函数内部调用带有 ISR
后缀的 FreeRTOS API)。回调函数需要返回一个布尔值,告诉 ISR 是否唤醒了高优先级任务。
I2C 主机事件回调函数列表见 i2c_master_event_callbacks_t
。
虽然 I2C 是一种同步通信协议,但也支持通过注册上述回调函数来实现异步行为,此时 I2C API 将成为非阻塞接口。但请注意,在同一总线上只有一个设备可以采用异步操作。
重要
I2C 主机异步传输仍然是一个实验性功能(问题在于当异步传输量过大时,会导致内存异常。)
i2c_master_event_callbacks_t::on_recv_done
可设置用于主机“传输完成”事件的回调函数。该函数原型在i2c_master_callback_t
中声明。
I2C 从机回调
当 I2C 从机总线触发中断时,将生成特定事件并通知 CPU。如果需要在发生这些事件时调用某些函数,可通过 i2c_slave_register_event_callbacks()
将这些函数挂接到中断服务程序 (ISR) 上。由于注册的回调函数是在中断上下文中被调用的,所以应确保这些函数不会导致延迟(例如,确保仅从函数中调用带有 ISR
后缀的 FreeRTOS API)。回调函数需要返回一个布尔值,告诉调用者是否唤醒了高优先级任务。
I2C 从机事件回调函数列表见 i2c_slave_event_callbacks_t
。
i2c_slave_event_callbacks_t::on_recv_done
可设置用于“接收完成”事件的回调函数。该函数原型在i2c_slave_received_callback_t
中声明。i2c_slave_event_callbacks_t::on_stretch_occur
可设置用于“时钟拉伸”事件的回调函数。该函数原型在i2c_slave_stretch_callback_t
中声明。
电源管理
如果控制器以 I2C_CLK_SRC_XTAL
为时钟源,则驱动程序不会为其安装电源管理锁,因为对于低功耗应用,只要时钟源能够提供足够的分辨率即可。
IRAM 安全
默认情况下,若 cache 因写入或擦除 flash 等原因而被禁用时,将推迟 I2C 中断。此时事件回调函数将无法按时执行,会影响实时应用的系统响应。
Kconfig 选项 CONFIG_I2C_ISR_IRAM_SAFE 能够做到以下几点:
即使 cache 被禁用,I2C 中断依旧正常运行。
将 ISR 使用的所有函数放入 IRAM 中。
将驱动程序对象放入 DRAM 中(以防它被意外映射到 PSRAM 中)。
启用以上选项,即使 cache 被禁用,I2C 中断依旧正常运行,但会增加 IRAM 的消耗。
线程安全
工厂函数 i2c_new_master_bus()
和 i2c_new_slave_device()
由驱动程序保证其线程安全,不需要额外的锁保护,也可从不同的 RTOS 任务中调用这些函数。应避免从多个任务中调用其他非线程安全的公共 I2C API,若确实需要调用,请添加额外的锁。
Kconfig 选项
CONFIG_I2C_ISR_IRAM_SAFE 将在 cache 被禁用时控制默认的 ISR 处理程序正常工作,详情请参阅 IRAM 安全。
CONFIG_I2C_ENABLE_DEBUG_LOG 可启用调试日志,但会增加固件二进制文件大小。
应用示例
peripherals/i2c/i2c_eeprom 通过读取和写入 I2C 连接的 EEPROM 展示了 I2C 驱动程序的使用方法。
peripherals/i2c/i2c_tools 基于 ESP32 控制台组件实现了一些 I2C 工具的基本功能。
API 参考
Header File
This header file can be included with:
#include "driver/i2c_master.h"
This header file is a part of the API provided by the
esp_driver_i2c
component. To declare that your component depends onesp_driver_i2c
, add the following to your CMakeLists.txt:REQUIRES esp_driver_i2c
or
PRIV_REQUIRES esp_driver_i2c
Functions
-
esp_err_t i2c_new_master_bus(const i2c_master_bus_config_t *bus_config, i2c_master_bus_handle_t *ret_bus_handle)
Allocate an I2C master bus.
- 参数
bus_config -- [in] I2C master bus configuration.
ret_bus_handle -- [out] I2C bus handle
- 返回
ESP_OK: I2C master bus initialized successfully.
ESP_ERR_INVALID_ARG: I2C bus initialization failed because of invalid argument.
ESP_ERR_NO_MEM: Create I2C bus failed because of out of memory.
ESP_ERR_NOT_FOUND: No more free bus.
-
esp_err_t i2c_master_bus_add_device(i2c_master_bus_handle_t bus_handle, const i2c_device_config_t *dev_config, i2c_master_dev_handle_t *ret_handle)
Add I2C master BUS device.
- 参数
bus_handle -- [in] I2C bus handle.
dev_config -- [in] device config.
ret_handle -- [out] device handle.
- 返回
ESP_OK: Create I2C master device successfully.
ESP_ERR_INVALID_ARG: I2C bus initialization failed because of invalid argument.
ESP_ERR_NO_MEM: Create I2C bus failed because of out of memory.
-
esp_err_t i2c_del_master_bus(i2c_master_bus_handle_t bus_handle)
Deinitialize the I2C master bus and delete the handle.
- 参数
bus_handle -- [in] I2C bus handle.
- 返回
ESP_OK: Delete I2C bus success, otherwise, failed.
Otherwise: Some module delete failed.
-
esp_err_t i2c_master_bus_rm_device(i2c_master_dev_handle_t handle)
I2C master bus delete device.
- 参数
handle -- i2c device handle
- 返回
ESP_OK: If device is successfully deleted.
-
esp_err_t i2c_master_transmit(i2c_master_dev_handle_t i2c_dev, const uint8_t *write_buffer, size_t write_size, int xfer_timeout_ms)
Perform a write transaction on the I2C bus. The transaction will be undergoing until it finishes or it reaches the timeout provided.
备注
If a callback was registered with
i2c_master_register_event_callbacks
, the transaction will be asynchronous, and thus, this function will return directly, without blocking. You will get finish information from callback. Besides, data buffer should always be completely prepared when callback is registered, otherwise, the data will get corrupt.- 参数
i2c_dev -- [in] I2C master device handle that created by
i2c_master_bus_add_device
.write_buffer -- [in] Data bytes to send on the I2C bus.
write_size -- [in] Size, in bytes, of the write buffer.
xfer_timeout_ms -- [in] Wait timeout, in ms. Note: -1 means wait forever.
- 返回
ESP_OK: I2C master transmit success
ESP_ERR_INVALID_ARG: I2C master transmit parameter invalid.
ESP_ERR_TIMEOUT: Operation timeout(larger than xfer_timeout_ms) because the bus is busy or hardware crash.
-
esp_err_t i2c_master_multi_buffer_transmit(i2c_master_dev_handle_t i2c_dev, i2c_master_transmit_multi_buffer_info_t *buffer_info_array, size_t array_size, int xfer_timeout_ms)
Transmit multiple buffers of data over an I2C bus.
This function transmits multiple buffers of data over an I2C bus using the specified I2C master device handle. It takes in an array of buffer information structures along with the size of the array and a transfer timeout value in milliseconds.
- 参数
i2c_dev -- I2C master device handle that created by
i2c_master_bus_add_device
.buffer_info_array -- Pointer to buffer information array.
array_size -- size of buffer information array.
xfer_timeout_ms -- Wait timeout, in ms. Note: -1 means wait forever.
- 返回
ESP_OK: I2C master transmit success
ESP_ERR_INVALID_ARG: I2C master transmit parameter invalid.
ESP_ERR_TIMEOUT: Operation timeout(larger than xfer_timeout_ms) because the bus is busy or hardware crash.
-
esp_err_t i2c_master_transmit_receive(i2c_master_dev_handle_t i2c_dev, const uint8_t *write_buffer, size_t write_size, uint8_t *read_buffer, size_t read_size, int xfer_timeout_ms)
Perform a write-read transaction on the I2C bus. The transaction will be undergoing until it finishes or it reaches the timeout provided.
备注
If a callback was registered with
i2c_master_register_event_callbacks
, the transaction will be asynchronous, and thus, this function will return directly, without blocking. You will get finish information from callback. Besides, data buffer should always be completely prepared when callback is registered, otherwise, the data will get corrupt.- 参数
i2c_dev -- [in] I2C master device handle that created by
i2c_master_bus_add_device
.write_buffer -- [in] Data bytes to send on the I2C bus.
write_size -- [in] Size, in bytes, of the write buffer.
read_buffer -- [out] Data bytes received from i2c bus.
read_size -- [in] Size, in bytes, of the read buffer.
xfer_timeout_ms -- [in] Wait timeout, in ms. Note: -1 means wait forever.
- 返回
ESP_OK: I2C master transmit-receive success
ESP_ERR_INVALID_ARG: I2C master transmit parameter invalid.
ESP_ERR_TIMEOUT: Operation timeout(larger than xfer_timeout_ms) because the bus is busy or hardware crash.
-
esp_err_t i2c_master_receive(i2c_master_dev_handle_t i2c_dev, uint8_t *read_buffer, size_t read_size, int xfer_timeout_ms)
Perform a read transaction on the I2C bus. The transaction will be undergoing until it finishes or it reaches the timeout provided.
备注
If a callback was registered with
i2c_master_register_event_callbacks
, the transaction will be asynchronous, and thus, this function will return directly, without blocking. You will get finish information from callback. Besides, data buffer should always be completely prepared when callback is registered, otherwise, the data will get corrupt.- 参数
i2c_dev -- [in] I2C master device handle that created by
i2c_master_bus_add_device
.read_buffer -- [out] Data bytes received from i2c bus.
read_size -- [in] Size, in bytes, of the read buffer.
xfer_timeout_ms -- [in] Wait timeout, in ms. Note: -1 means wait forever.
- 返回
ESP_OK: I2C master receive success
ESP_ERR_INVALID_ARG: I2C master receive parameter invalid.
ESP_ERR_TIMEOUT: Operation timeout(larger than xfer_timeout_ms) because the bus is busy or hardware crash.
-
esp_err_t i2c_master_probe(i2c_master_bus_handle_t bus_handle, uint16_t address, int xfer_timeout_ms)
Probe I2C address, if address is correct and ACK is received, this function will return ESP_OK.
- Attention
Pull-ups must be connected to the SCL and SDA pins when this function is called. If you get
ESP_ERR_TIMEOUT while
xfer_timeout_mswas parsed correctly, you should check the pull-up resistors. If you do not have proper resistors nearby.
flags.enable_internal_pullup` is also acceptable.
备注
The principle of this function is to sent device address with a write command. If the device on your I2C bus, there would be an ACK signal and function returns
ESP_OK
. If the device is not on your I2C bus, there would be a NACK signal and function returnsESP_ERR_NOT_FOUND
.ESP_ERR_TIMEOUT
is not an expected failure, which indicated that the i2c probe not works properly, usually caused by pull-up resistors not be connected properly. Suggestion check data on SDA/SCL line to see whether there is ACK/NACK signal is on line when i2c probe function fails.备注
There are lots of I2C devices all over the world, we assume that not all I2C device support the behavior like
device_address+nack/ack
. So, if the on line data is strange and no ack/nack got respond. Please check the device datasheet.- 参数
bus_handle -- [in] I2C master device handle that created by
i2c_master_bus_add_device
.address -- [in] I2C device address that you want to probe.
xfer_timeout_ms -- [in] Wait timeout, in ms. Note: -1 means wait forever (Not recommended in this function).
- 返回
ESP_OK: I2C device probe successfully
ESP_ERR_NOT_FOUND: I2C probe failed, doesn't find the device with specific address you gave.
ESP_ERR_TIMEOUT: Operation timeout(larger than xfer_timeout_ms) because the bus is busy or hardware crash.
-
esp_err_t i2c_master_register_event_callbacks(i2c_master_dev_handle_t i2c_dev, const i2c_master_event_callbacks_t *cbs, void *user_data)
Register I2C transaction callbacks for a master device.
备注
User can deregister a previously registered callback by calling this function and setting the callback member in the
cbs
structure to NULL.备注
When CONFIG_I2C_ISR_IRAM_SAFE is enabled, the callback itself and functions called by it should be placed in IRAM. The variables used in the function should be in the SRAM as well. The
user_data
should also reside in SRAM.备注
If the callback is used for helping asynchronous transaction. On the same bus, only one device can be used for performing asynchronous operation.
- 参数
i2c_dev -- [in] I2C master device handle that created by
i2c_master_bus_add_device
.cbs -- [in] Group of callback functions
user_data -- [in] User data, which will be passed to callback functions directly
- 返回
ESP_OK: Set I2C transaction callbacks successfully
ESP_ERR_INVALID_ARG: Set I2C transaction callbacks failed because of invalid argument
ESP_FAIL: Set I2C transaction callbacks failed because of other error
-
esp_err_t i2c_master_bus_reset(i2c_master_bus_handle_t bus_handle)
Reset the I2C master bus.
- 参数
bus_handle -- I2C bus handle.
- 返回
ESP_OK: Reset succeed.
ESP_ERR_INVALID_ARG: I2C master bus handle is not initialized.
Otherwise: Reset failed.
-
esp_err_t i2c_master_bus_wait_all_done(i2c_master_bus_handle_t bus_handle, int timeout_ms)
Wait for all pending I2C transactions done.
- 参数
bus_handle -- [in] I2C bus handle
timeout_ms -- [in] Wait timeout, in ms. Specially, -1 means to wait forever.
- 返回
ESP_OK: Flush transactions successfully
ESP_ERR_INVALID_ARG: Flush transactions failed because of invalid argument
ESP_ERR_TIMEOUT: Flush transactions failed because of timeout
ESP_FAIL: Flush transactions failed because of other error
Structures
-
struct i2c_master_bus_config_t
I2C master bus specific configurations.
Public Members
-
i2c_port_num_t i2c_port
I2C port number,
-1
for auto selecting, (not include LP I2C instance)
-
gpio_num_t sda_io_num
GPIO number of I2C SDA signal, pulled-up internally
-
gpio_num_t scl_io_num
GPIO number of I2C SCL signal, pulled-up internally
-
i2c_clock_source_t clk_source
Clock source of I2C master bus
-
uint8_t glitch_ignore_cnt
If the glitch period on the line is less than this value, it can be filtered out, typically value is 7 (unit: I2C module clock cycle)
-
int intr_priority
I2C interrupt priority, if set to 0, driver will select the default priority (1,2,3).
-
size_t trans_queue_depth
Depth of internal transfer queue, increase this value can support more transfers pending in the background, only valid in asynchronous transaction. (Typically max_device_num * per_transaction)
-
uint32_t enable_internal_pullup
Enable internal pullups. Note: This is not strong enough to pullup buses under high-speed frequency. Recommend proper external pull-up if possible
-
struct i2c_master_bus_config_t::[anonymous] flags
I2C master config flags
-
i2c_port_num_t i2c_port
-
struct i2c_device_config_t
I2C device configuration.
Public Members
-
i2c_addr_bit_len_t dev_addr_length
Select the address length of the slave device.
-
uint16_t device_address
I2C device raw address. (The 7/10 bit address without read/write bit)
-
uint32_t scl_speed_hz
I2C SCL line frequency.
-
uint32_t scl_wait_us
Timeout value. (unit: us). Please note this value should not be so small that it can handle stretch/disturbance properly. If 0 is set, that means use the default reg value
-
uint32_t disable_ack_check
Disable ACK check. If this is set false, that means ack check is enabled, the transaction will be stopped and API returns error when nack is detected.
-
struct i2c_device_config_t::[anonymous] flags
I2C device config flags
-
i2c_addr_bit_len_t dev_addr_length
-
struct i2c_master_transmit_multi_buffer_info_t
I2C master transmit buffer information structure.
-
struct i2c_master_event_callbacks_t
Group of I2C master callbacks, can be used to get status during transaction or doing other small things. But take care potential concurrency issues.
备注
The callbacks are all running under ISR context
备注
When CONFIG_I2C_ISR_IRAM_SAFE is enabled, the callback itself and functions called by it should be placed in IRAM. The variables used in the function should be in the SRAM as well.
Public Members
-
i2c_master_callback_t on_trans_done
I2C master transaction finish callback
-
i2c_master_callback_t on_trans_done
Header File
This header file can be included with:
#include "driver/i2c_slave.h"
This header file is a part of the API provided by the
esp_driver_i2c
component. To declare that your component depends onesp_driver_i2c
, add the following to your CMakeLists.txt:REQUIRES esp_driver_i2c
or
PRIV_REQUIRES esp_driver_i2c
Functions
-
esp_err_t i2c_new_slave_device(const i2c_slave_config_t *slave_config, i2c_slave_dev_handle_t *ret_handle)
Initialize an I2C slave device.
- 参数
slave_config -- [in] I2C slave device configurations
ret_handle -- [out] Return a generic I2C device handle
- 返回
ESP_OK: I2C slave device initialized successfully
ESP_ERR_INVALID_ARG: I2C device initialization failed because of invalid argument.
ESP_ERR_NO_MEM: Create I2C device failed because of out of memory.
-
esp_err_t i2c_del_slave_device(i2c_slave_dev_handle_t i2c_slave)
Deinitialize the I2C slave device.
- 参数
i2c_slave -- [in] I2C slave device handle that created by
i2c_new_slave_device
.- 返回
ESP_OK: Delete I2C device successfully.
ESP_ERR_INVALID_ARG: I2C device initialization failed because of invalid argument.
-
esp_err_t i2c_slave_receive(i2c_slave_dev_handle_t i2c_slave, uint8_t *data, size_t buffer_size)
Read bytes from I2C internal buffer. Start a job to receive I2C data.
备注
This function is non-blocking, it initiates a new receive job and then returns. User should check the received data from the
on_recv_done
callback that registered byi2c_slave_register_event_callbacks()
.- 参数
i2c_slave -- [in] I2C slave device handle that created by
i2c_new_slave_device
.data -- [out] Buffer to store data from I2C fifo. Should be valid until
on_recv_done
is triggered.buffer_size -- [in] Buffer size of data that provided by users.
- 返回
ESP_OK: I2C slave receive success.
ESP_ERR_INVALID_ARG: I2C slave receive parameter invalid.
ESP_ERR_NOT_SUPPORTED: This function should be work in fifo mode, but I2C_SLAVE_NONFIFO mode is configured
-
esp_err_t i2c_slave_transmit(i2c_slave_dev_handle_t i2c_slave, const uint8_t *data, int size, int xfer_timeout_ms)
Write bytes to internal ringbuffer of the I2C slave data. When the TX fifo empty, the ISR will fill the hardware FIFO with the internal ringbuffer's data.
备注
If you connect this slave device to some master device, the data transaction direction is from slave device to master device.
- 参数
i2c_slave -- [in] I2C slave device handle that created by
i2c_new_slave_device
.data -- [in] Buffer to write to slave fifo, can pickup by master. Can be freed after this function returns. Equal or larger than
size
.size -- [in] In bytes, of
data
buffer.xfer_timeout_ms -- [in] Wait timeout, in ms. Note: -1 means wait forever.
- 返回
ESP_OK: I2C slave transmit success.
ESP_ERR_INVALID_ARG: I2C slave transmit parameter invalid.
ESP_ERR_TIMEOUT: Operation timeout(larger than xfer_timeout_ms) because the device is busy or hardware crash.
ESP_ERR_NOT_SUPPORTED: This function should be work in fifo mode, but I2C_SLAVE_NONFIFO mode is configured
-
esp_err_t i2c_slave_register_event_callbacks(i2c_slave_dev_handle_t i2c_slave, const i2c_slave_event_callbacks_t *cbs, void *user_data)
Set I2C slave event callbacks for I2C slave channel.
备注
User can deregister a previously registered callback by calling this function and setting the callback member in the
cbs
structure to NULL.备注
When CONFIG_I2C_ISR_IRAM_SAFE is enabled, the callback itself and functions called by it should be placed in IRAM. The variables used in the function should be in the SRAM as well. The
user_data
should also reside in SRAM.- 参数
i2c_slave -- [in] I2C slave device handle that created by
i2c_new_slave_device
.cbs -- [in] Group of callback functions
user_data -- [in] User data, which will be passed to callback functions directly
- 返回
ESP_OK: Set I2C transaction callbacks successfully
ESP_ERR_INVALID_ARG: Set I2C transaction callbacks failed because of invalid argument
ESP_FAIL: Set I2C transaction callbacks failed because of other error
-
esp_err_t i2c_slave_read_ram(i2c_slave_dev_handle_t i2c_slave, uint8_t ram_address, uint8_t *data, size_t receive_size)
Read bytes from I2C internal ram. This can be only used when
access_ram_en
in configuration structure set to true.- 参数
i2c_slave -- [in] I2C slave device handle that created by
i2c_new_slave_device
.ram_address -- [in] The offset of RAM (Cannot larger than I2C RAM memory)
data -- [out] Buffer to store data read from I2C ram.
receive_size -- [in] Received size from RAM.
- 返回
ESP_OK: I2C slave transmit success.
ESP_ERR_INVALID_ARG: I2C slave transmit parameter invalid.
ESP_ERR_NOT_SUPPORTED: This function should be work in non-fifo mode, but I2C_SLAVE_FIFO mode is configured
-
esp_err_t i2c_slave_write_ram(i2c_slave_dev_handle_t i2c_slave, uint8_t ram_address, const uint8_t *data, size_t size)
Write bytes to I2C internal ram. This can be only used when
access_ram_en
in configuration structure set to true.- 参数
i2c_slave -- [in] I2C slave device handle that created by
i2c_new_slave_device
.ram_address -- [in] The offset of RAM (Cannot larger than I2C RAM memory)
data -- [in] Buffer to fill.
size -- [in] Received size from RAM.
- 返回
ESP_OK: I2C slave transmit success.
ESP_ERR_INVALID_ARG: I2C slave transmit parameter invalid.
ESP_ERR_INVALID_SIZE: Write size is larger than
ESP_ERR_NOT_SUPPORTED: This function should be work in non-fifo mode, but I2C_SLAVE_FIFO mode is configured
Structures
-
struct i2c_slave_config_t
I2C slave specific configurations.
Public Members
-
i2c_port_num_t i2c_port
I2C port number,
-1
for auto selecting
-
gpio_num_t sda_io_num
SDA IO number used by I2C bus
-
gpio_num_t scl_io_num
SCL IO number used by I2C bus
-
i2c_clock_source_t clk_source
Clock source of I2C bus.
-
uint32_t send_buf_depth
Depth of internal transfer ringbuffer, increase this value can support more transfers pending in the background
-
uint16_t slave_addr
I2C slave address
-
i2c_addr_bit_len_t addr_bit_len
I2C slave address in bit length
-
int intr_priority
I2C interrupt priority, if set to 0, driver will select the default priority (1,2,3).
-
uint32_t stretch_en
Enable slave stretch
-
uint32_t broadcast_en
I2C slave enable broadcast
-
uint32_t access_ram_en
Can get access to I2C RAM directly
-
struct i2c_slave_config_t::[anonymous] flags
I2C slave config flags
-
i2c_port_num_t i2c_port
-
struct i2c_slave_event_callbacks_t
Group of I2C slave callbacks (e.g. get i2c slave stretch cause). But take care of potential concurrency issues.
备注
The callbacks are all running under ISR context
备注
When CONFIG_I2C_ISR_IRAM_SAFE is enabled, the callback itself and functions called by it should be placed in IRAM. The variables used in the function should be in the SRAM as well.
Public Members
-
i2c_slave_stretch_callback_t on_stretch_occur
I2C slave stretched callback
-
i2c_slave_received_callback_t on_recv_done
I2C slave receive done callback
-
i2c_slave_stretch_callback_t on_stretch_occur
Header File
This header file can be included with:
#include "driver/i2c_types.h"
This header file is a part of the API provided by the
esp_driver_i2c
component. To declare that your component depends onesp_driver_i2c
, add the following to your CMakeLists.txt:REQUIRES esp_driver_i2c
or
PRIV_REQUIRES esp_driver_i2c
Structures
-
struct i2c_master_event_data_t
Data type used in I2C event callback.
Public Members
-
i2c_master_event_t event
The I2C hardware event that I2C callback is called.
-
i2c_master_event_t event
-
struct i2c_slave_rx_done_event_data_t
Event structure used in I2C slave.
Public Members
-
uint8_t *buffer
Pointer for buffer received in callback.
-
uint8_t *buffer
-
struct i2c_slave_stretch_event_data_t
Stretch cause event structure used in I2C slave.
Public Members
-
i2c_slave_stretch_cause_t stretch_cause
Stretch cause can be got in callback
-
i2c_slave_stretch_cause_t stretch_cause
Type Definitions
-
typedef int i2c_port_num_t
I2C port number.
-
typedef struct i2c_master_bus_t *i2c_master_bus_handle_t
Type of I2C master bus handle.
-
typedef struct i2c_master_dev_t *i2c_master_dev_handle_t
Type of I2C master bus device handle.
-
typedef struct i2c_slave_dev_t *i2c_slave_dev_handle_t
Type of I2C slave device handle.
-
typedef bool (*i2c_master_callback_t)(i2c_master_dev_handle_t i2c_dev, const i2c_master_event_data_t *evt_data, void *arg)
An callback for I2C transaction.
- Param i2c_dev
[in] Handle for I2C device.
- Param evt_data
[out] I2C capture event data, fed by driver
- Param arg
[in] User data, set in
i2c_master_register_event_callbacks()
- Return
Whether a high priority task has been waken up by this function
-
typedef bool (*i2c_slave_received_callback_t)(i2c_slave_dev_handle_t i2c_slave, const i2c_slave_rx_done_event_data_t *evt_data, void *arg)
Callback signature for I2C slave.
- Param i2c_slave
[in] Handle for I2C slave.
- Param evt_data
[out] I2C capture event data, fed by driver
- Param arg
[in] User data, set in
i2c_slave_register_event_callbacks()
- Return
Whether a high priority task has been waken up by this function
-
typedef bool (*i2c_slave_stretch_callback_t)(i2c_slave_dev_handle_t i2c_slave, const i2c_slave_stretch_event_data_t *evt_cause, void *arg)
Callback signature for I2C slave stretch.
- Param i2c_slave
[in] Handle for I2C slave.
- Param evt_cause
[out] I2C capture event cause, fed by driver
- Param arg
[in] User data, set in
i2c_slave_register_event_callbacks()
- Return
Whether a high priority task has been waken up by this function
Enumerations
-
enum i2c_master_status_t
Enumeration for I2C fsm status.
Values:
-
enumerator I2C_STATUS_READ
read status for current master command
-
enumerator I2C_STATUS_WRITE
write status for current master command
-
enumerator I2C_STATUS_START
Start status for current master command
-
enumerator I2C_STATUS_STOP
stop status for current master command
-
enumerator I2C_STATUS_IDLE
idle status for current master command
-
enumerator I2C_STATUS_ACK_ERROR
ack error status for current master command
-
enumerator I2C_STATUS_DONE
I2C command done
-
enumerator I2C_STATUS_TIMEOUT
I2C bus status error, and operation timeout
-
enumerator I2C_STATUS_READ
Header File
This header file can be included with:
#include "hal/i2c_types.h"
Structures
-
struct i2c_hal_clk_config_t
Data structure for calculating I2C bus timing.
Public Members
-
uint16_t clkm_div
I2C core clock divider
-
uint16_t scl_low
I2C scl low period
-
uint16_t scl_high
I2C scl high period
-
uint16_t scl_wait_high
I2C scl wait_high period
-
uint16_t sda_hold
I2C scl low period
-
uint16_t sda_sample
I2C sda sample time
-
uint16_t setup
I2C start and stop condition setup period
-
uint16_t hold
I2C start and stop condition hold period
-
uint16_t tout
I2C bus timeout period
-
uint16_t clkm_div
Type Definitions
-
typedef soc_periph_i2c_clk_src_t i2c_clock_source_t
I2C group clock source.
Enumerations
-
enum i2c_port_t
I2C port number, can be I2C_NUM_0 ~ (I2C_NUM_MAX-1).
Values:
-
enumerator I2C_NUM_0
I2C port 0
-
enumerator I2C_NUM_MAX
I2C port max
-
enumerator I2C_NUM_0
-
enum i2c_addr_bit_len_t
Enumeration for I2C device address bit length.
Values:
-
enumerator I2C_ADDR_BIT_LEN_7
i2c address bit length 7
-
enumerator I2C_ADDR_BIT_LEN_10
i2c address bit length 10
-
enumerator I2C_ADDR_BIT_LEN_7
-
enum i2c_mode_t
Values:
-
enumerator I2C_MODE_SLAVE
I2C slave mode
-
enumerator I2C_MODE_MASTER
I2C master mode
-
enumerator I2C_MODE_MAX
-
enumerator I2C_MODE_SLAVE
-
enum i2c_rw_t
Values:
-
enumerator I2C_MASTER_WRITE
I2C write data
-
enumerator I2C_MASTER_READ
I2C read data
-
enumerator I2C_MASTER_WRITE
-
enum i2c_trans_mode_t
Values:
-
enumerator I2C_DATA_MODE_MSB_FIRST
I2C data msb first
-
enumerator I2C_DATA_MODE_LSB_FIRST
I2C data lsb first
-
enumerator I2C_DATA_MODE_MAX
-
enumerator I2C_DATA_MODE_MSB_FIRST
-
enum i2c_addr_mode_t
Values:
-
enumerator I2C_ADDR_BIT_7
I2C 7bit address for slave mode
-
enumerator I2C_ADDR_BIT_10
I2C 10bit address for slave mode
-
enumerator I2C_ADDR_BIT_MAX
-
enumerator I2C_ADDR_BIT_7
-
enum i2c_ack_type_t
Values:
-
enumerator I2C_MASTER_ACK
I2C ack for each byte read
-
enumerator I2C_MASTER_NACK
I2C nack for each byte read
-
enumerator I2C_MASTER_LAST_NACK
I2C nack for the last byte
-
enumerator I2C_MASTER_ACK_MAX
-
enumerator I2C_MASTER_ACK
-
enum i2c_slave_stretch_cause_t
Enum for I2C slave stretch causes.
Values:
-
enumerator I2C_SLAVE_STRETCH_CAUSE_ADDRESS_MATCH
Stretching SCL low when the slave is read by the master and the address just matched
-
enumerator I2C_SLAVE_STRETCH_CAUSE_TX_EMPTY
Stretching SCL low when TX FIFO is empty in slave mode
-
enumerator I2C_SLAVE_STRETCH_CAUSE_RX_FULL
Stretching SCL low when RX FIFO is full in slave mode
-
enumerator I2C_SLAVE_STRETCH_CAUSE_SENDING_ACK
Stretching SCL low when slave sending ACK
-
enumerator I2C_SLAVE_STRETCH_CAUSE_ADDRESS_MATCH