Downmix
This API is intended for mixing of two audio files (streams), defined as the base audio file and the newcome audio file, into one output audio file.
The newcome audio file will be downmixed into the base audio file with individual gains applied to each file.
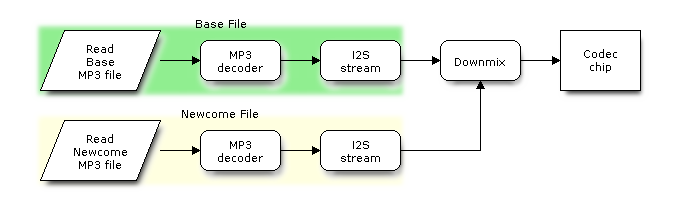
Illustration of Downmixing Process
The number of channel(s) of the output audio file will be the same with that of the base audio file. The number of channel(s) of the newcome audio file will also be changed to the same with the base audio file, if it is different from that of the base audio file.
The downmix process has 3 states:
Bypass Downmixing – Only the base audio file will be processed;
Switch on Downmixing – The base audio file and the target audio file will first enter the transition period, during which the gains of these two files will be changed from the original level to the target level; then enter the stable period, sharing a same target gain;
Switch off Downmixing – The base audio file and the target audio file will first enter the transition period, during which the gains of these two files will be changed back to their original levels; then enter the stable period, with their original gains, respectively. After that, the downmix process enters the bypass state.
Note that, the sample rates of the base audio file and the newcome audio file must be the same, otherwise an error occurs.
Application Example
Implementation of this API is demonstrated in advanced_examples/downmix_pipeline example.
API Reference
Header File
Functions
-
void downmix_set_input_rb_timeout(audio_element_handle_t self, int ticks_to_wait, int index)
Sets the downmix timeout.
- Parameters
self – Audio element handle
ticks_to_wait – Input ringbuffer timeout
index – The index of multi input ringbuffer
-
void downmix_set_input_rb(audio_element_handle_t self, ringbuf_handle_t rb, int index)
Sets the downmix input ringbuffer. refer to
ringbuf.h
- Parameters
self – Audio element handle
rb – Handle of ringbuffer
index – The index of multi input ringbuffer.
-
esp_err_t downmix_set_output_type(audio_element_handle_t self, esp_downmix_output_type_t output_type)
Set channel mode of output data. Only supported mono and dual.
- Parameters
self – Audio element handle
output_type – Down-mixer output type.
- Returns
ESP_OK on success
ESP_ERR_INVALID_ARG invalid arguments
-
esp_err_t downmix_set_work_mode(audio_element_handle_t self, esp_downmix_work_mode_t mode)
Sets BYPASS, ON or OFF status of down-mixer.
- Parameters
self – Audio element handle
mode – Down-mixer work mode.
- Returns
ESP_OK on success
ESP_ERR_INVALID_ARG invalid arguments
-
esp_err_t downmix_set_out_ctx_info(audio_element_handle_t self, esp_downmix_out_ctx_type_t out_ctx)
Passes content of per channel output stream by down-mixer.
- Parameters
self – Audio element handle
out_ctx – Content of output stream.
- Returns
ESP_OK on success
ESP_ERR_INVALID_ARG invalid arguments
-
esp_err_t downmix_set_source_stream_info(audio_element_handle_t self, int rate, int ch, int index)
Sets the sample rate and the number of channels of input stream to be processed.
- Parameters
self – Audio element handle
rate – Sample rate of the input stream
ch – Channel number of the input stream. Only supported mono and dual.
index – The index of input stream. The index must be in [0, SOURCE_NUM_MAX - 1] range.
- Returns
ESP_OK on success
ESP_ERR_INVALID_ARG invalid arguments
-
esp_err_t downmix_set_gain_info(audio_element_handle_t self, float *gain, int index)
Sets the audio gain to be processed.
- Parameters
self – Audio element handle
gain – The gain array of downmix which the array size is 2 and the gain data range is [-100, 100], unit: dB.
index – The index of input stream. The index must be in [0, SOURCE_NUM_MAX - 1] range.
- Returns
ESP_OK on success
ESP_ERR_INVALID_ARG invalid arguments
-
esp_err_t downmix_set_transit_time_info(audio_element_handle_t self, int transit_time, int index)
Sets the audio
transit_time
to be processed.- Parameters
self – Audio element handle
transit_time – The reset value of
transit_time
index – The index of input stream. The index must be in [0, SOURCE_NUM_MAX - 1] range
- Returns
ESP_OK on success
ESP_ERR_INVALID_ARG invalid arguments
-
esp_err_t source_info_init(audio_element_handle_t self, esp_downmix_input_info_t *source_info)
Initializes information of the source streams for downmixing.
- Parameters
self – Audio element handle
source_info – The information array of source streams
- Returns
ESP_OK on success
ESP_ERR_INVALID_ARG invalid arguments
-
audio_element_handle_t downmix_init(downmix_cfg_t *config)
Initializes the Audio Element handle for downmixing.
- Parameters
config – the configuration
- Returns
The audio element handler
Structures
-
struct downmix_cfg_t
Downmix configuration.
Public Members
-
esp_downmix_info_t downmix_info
Downmix information
-
int max_sample
The number of samples per downmix processing
-
int out_rb_size
Size of ring buffer
-
int task_stack
Size of task stack
-
int task_core
Task running in core…
-
int task_prio
Task priority (based on the FreeRTOS priority)
-
bool stack_in_ext
Try to allocate stack in external memory
-
esp_downmix_info_t downmix_info
Macros
-
DOWNMIX_TASK_STACK
-
DOWNMIX_TASK_CORE
-
DOWNMIX_TASK_PRIO
-
DOWNMIX_RINGBUFFER_SIZE
-
DM_BUF_SIZE
-
DEFAULT_DOWNMIX_CONFIG()