LCD Development Guide
This guide mainly contains the following sections:
Supported Interface Types: Espressif’s support for different LCD interfaces in each series of chips.
Driver and Examples: LCD driver and examples provided by Espressif Systems.
Development Framework: Develop software and hardware framework for LCD.
Development Steps: Detailed steps for developing LCD applications.
Common Problems: Lists common problems in developing LCD applications.
Related Documents: Links to relevant documentation are listed.
Terminology
Please refer to the LCD Terms Table 。
Supported Interface Types
Espressif Systems provides comprehensive support for all interface types introduced in the LCD Overview - Driver Interface. Specific support for each series of ESP chips within this section is as follows:
Soc |
SPI(QSPI) |
I80 |
RGB |
MIPI-DSI |
---|---|---|---|---|
ESP32 |
||||
ESP32-C3 |
||||
ESP32-C6 |
||||
ESP32-S2 |
||||
ESP32-S3 |
||||
ESP32-P4 |
Driver and Examples
LCD Peripheral Driver is located in the components/esp_lcd <https://docs.espressif.com/projects/esp-idf/en/latest/esp32s3/api-reference/peripherals/lcd.html>_ directory under ESP-IDF. Currently, it supports the I2C
, SPI (QSPI)
, I80
, and RGB
interfaces. The following table lists the LCD driver components officially ported by Espressif based on esp_lcd, and these components will continue to be updated:
接口 |
LCD 控制器 |
---|---|
I2C |
|
SPI |
axs15231b, st7789, nt35510, gc9b71, nv3022b, sh8601, spd2010, st77916, st77922, gc9a01, ili9341, ssd1681, st7796 |
QSPI |
axs15231b, gc9b71, sh8601, spd2010, st77903, st77916, st77922 |
I80 |
|
MIPI-DSI |
ek79007, jd9165, jd9365, st7701, st7703, st77922, ili9881c, hx8399 |
3-wire SPI + RGB |
Please note:
The st7789, nt35510, and ssd1306 components are maintained in the ESP-IDF. Other components can be found in the Espressif IDF Component Registry.
Although the models of the LCD driver IC might be identical, different screens require specific configurations through initialization commands provided by their respective manufacturers. Most driver components support the customization of initialization commands during the initialization of the LCD device. If it is not supported, please refer to the method.
LCD Example is located in the examples/peripherals/lcd directory under ESP-IDF and the examples/display/lcd directory under esp-iot-solution. These serve as a reference for the usage of the LCD driver component.
Note
It is recommended to develop based on ESP-IDF release/v5.1 and above version branches, because lower versions may lack to support some parts of the important new features, especially for the
RGB
interface.For LCDs using the
3-wire SPI + RGB
interface, please refer to the example esp_lcd_st7701 - Example use.
Development Framework
Hardware Framework
For SPI/I80 LCD, ESP can send commands to configure the LCD and transmit regional color data to refresh the screen through a single peripheral interface. The LCD driver IC will store the received color data in full-screen size GRAM and display full-screen color data on the panel at a fixed refresh rate. Importantly, these two processes are performed in asynchronously. The schematic diagram below illustrates the hardware driver framework of SPI/I80 LCD:
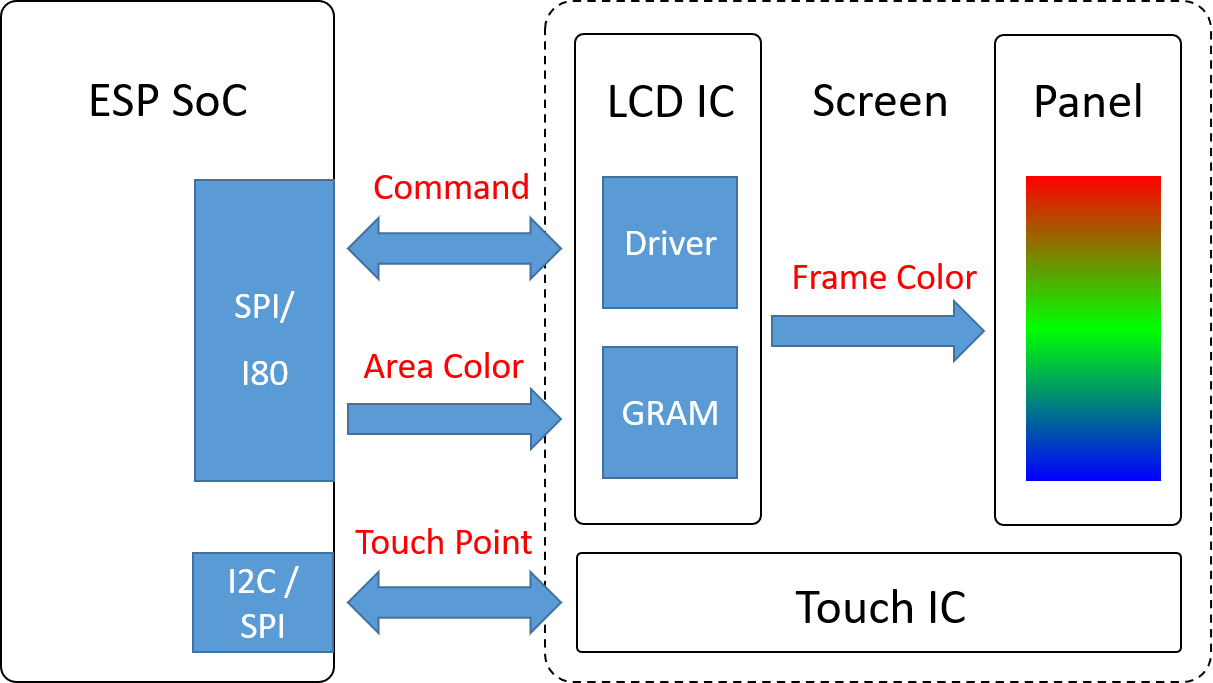
Schematic diagram of hardware driver framework - SPI/I80 LCD
For most RGB LCDs, the ESP needs to use two different interfaces. On one hand, it utilizes the 3-wire SPI interface to send commands for configuring the LCD. On the other hand, it uses the RGB interface to transmit full-screen color data for screen refresh. Since the LCD’s driver IC does not have a built-in Graphic RAM (GRAM), it directly displays the received color data on the panel, making these two processes synchronous. The following is a schematic diagram of the hardware driving framework for RGB LCDs:

Schematic diagram of hardware driver framework - RGB LCD
By comparing these two frameworks, it can be observed that, in contrast to SPI/I80 LCDs, RGB LCDs not only require the ESP to use two interfaces for transmitting commands and color data separately but also require that the ESP provides a full-screen-sized Graphic RAM (GRAM) for screen refresh. Due to the limited space in the on-chip SRAM, GRAM is typically placed in the PSRAM.
For QSPI LCDs, different models of driver ICs may require different driving methods. For example, the SPD2010 IC has a built-in GRAM, and its driving method is similar to SPI/I80 LCDs. On the other hand, the ST77903 IC does not have internal GRAM, and its driving method is similar to RGB LCDs. However, both of them use a single peripheral interface to transmit commands and color data. Below are schematic diagrams illustrating the hardware driving frameworks for these two types of QSPI LCDs:
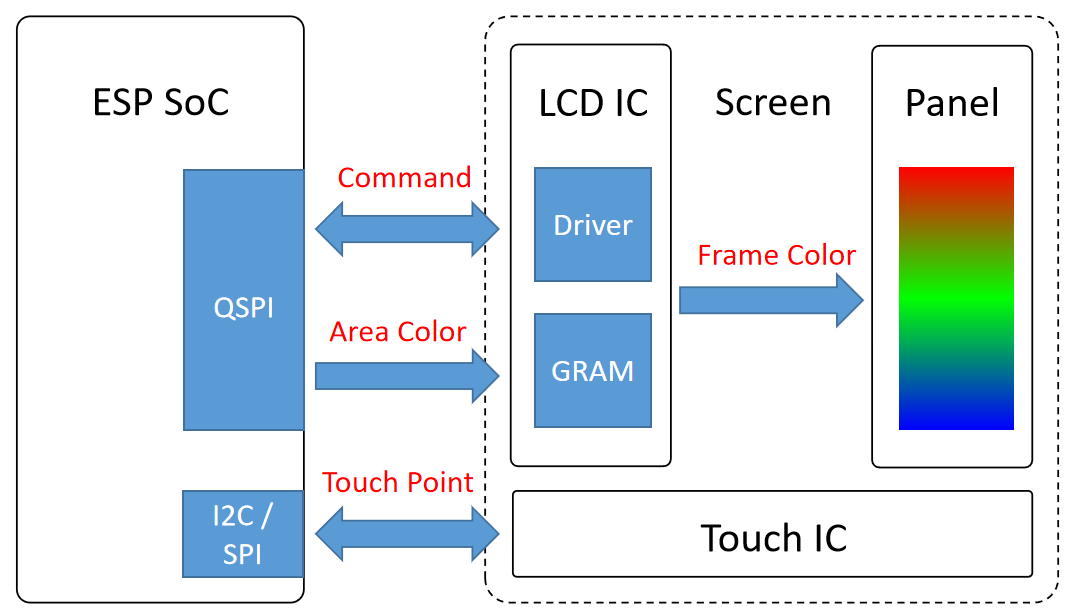
Schematic diagram of hardware driver framework - QSPI LCD (with GRAM)
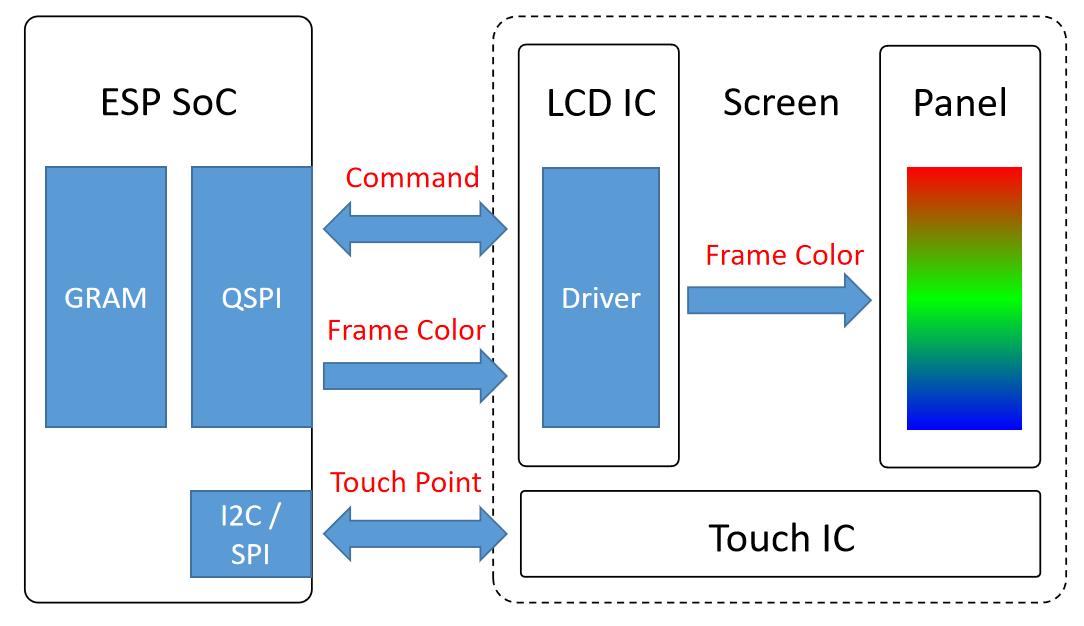
Schematic diagram of hardware driver framework - QSPI LCD (without GRAM)
Software Framework
The software development framework primarily consists of three layers: SDK (Software Development Kit), Driver, and APP (Application).
SDK layer: ESP-IDF serves as the foundational element of the framework. It not only includes
I2C
,SPI (QSPI)
,I80
andRGB
required to drive LCD and other peripherals, it also provides unified APIs through theesp_lcd
component to operate the interface and LCD, such as command and parameter transmission, LCD image refresh, inversion, mirroring and other functions.Driver layer: Based on the APIs provided by the SDK, various device drivers can be implemented, and the porting of LVGL (GUI framework) can be implemented by initializing interface devices and LCD devices.
APP layer: Use the APIs provided by LVGL to implement various GUI functions, such as displaying pictures, animations, text, etc.
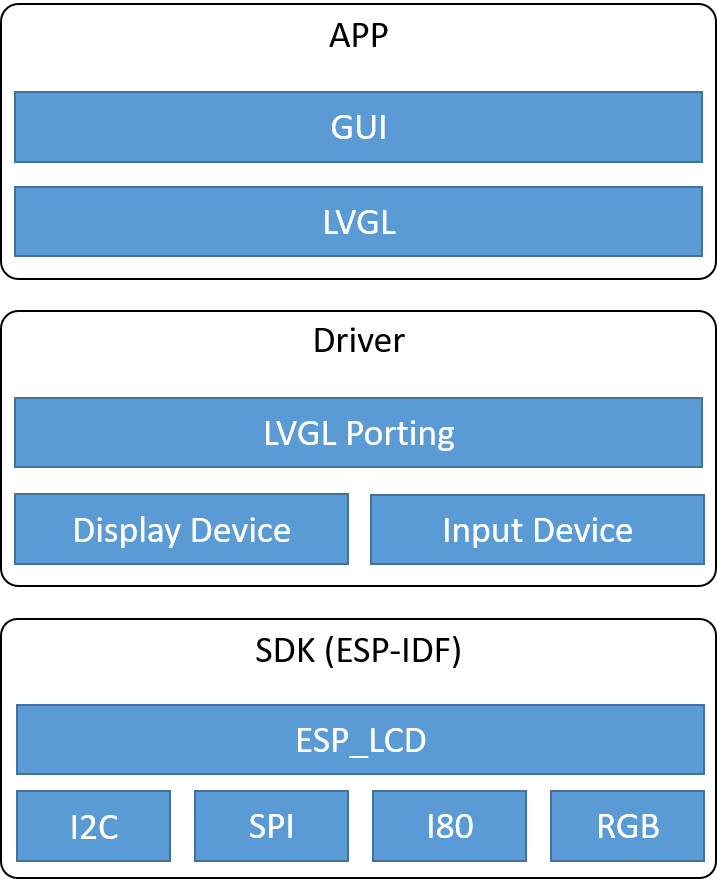
Schematic diagram of software development framework
Development Steps
Initialize interface device
First, initialize the peripherals corresponding to the LCD interface. Then, create the interface device and get its handle, the data type of the handle should be esp_lcd_panel_io_handle_t
. In this way, unified interface common APIs can be used for data transmission.
Note
For LCDs that only use the RGB
interface, there is no need to create its interface device, please refer directly to LCD Initialization.
Different types of LCD interfaces require the use of different peripherals. The following describes the device initialization process of several common interfaces:
I80 LCD Introduction - Initialization interface device (to be updated)
QSPI LCD Introduction - Initializing interface devices (to be updated)
For a more detailed description of this part, please refer to ESP-IDF Programming Guide.
Initialize LCD device
Since different models of LCD driver ICs may have different commands (registers) and parameters, and different interface types may also use different data formats and driving methods, here first need to use interface common APIs for specific interfaces to port the target LCD driver, then create the LCD device and obtain the data type esp_lcd_panel_handle_t
handle, ultimately enabling applications to pass unified LCD common APIs to operate the LCD device.
Note
For LCDs that only use the RGB
interface, there is no need to port its driver components. Please refer directly to LCD Initialization.
Before porting the driver component, please first try to obtain the components of the target LCD driver IC directly from LCD driver component. If the component does not exist, it can also be porting based on an existing component with the same interface type. LCD drivers with different interface types may have different porting principles. The following describes the porting methods of LCD driver components with several common interfaces:
I80 LCD Introduction - Porting driver component (to be updated)
QSPI LCD Introduction - Porting driver component (to be updated)
Then, the LCD initialization can be realized by using the driver component. The LCD initialization of several common interfaces is explained below:
I80 LCD Introduction - Initialize LCD device (To be updated)
QSPI LCD Introduction - Initialize LCD device (To be updated)
For a more detailed description of this part, please refer to the ESP-IDF Programming Guide.
Porting LVGL
(To be updated)
Design GUI
(To be updated)
Common Problems
The following lists some common issues encountered during the development of LCD applications. Please click on the issues to navigate and view the solutions.